The plugin Advanced Custom Fields in its Pro version comes with the option to add a “Repeater Field.” The Repeater Field allows the user to add an unlimited number of rows to a post or page in WordPress.
This field can be an ideal solution for a client who wants to add content or images to a page without touching the code. In truth, there is no site I’ve developed in recent years that hasn’t used such a Repeater Field.
In this guide, we will use the same Repeater Field to create a Questions and Answers page in WordPress (FAQ Page), that will look similar to the following FAQ component:
Start with Advanced Custom Fields Settings
Download and install the ACF Pro plugin (the Pro version is paid). Install it and then create a page named Questions and Answers as you would create any page in WordPress.
Now, go to ACF and create a new field group named FAQs. Within this group, create a Repeater Field and name it faqs
. Then, add two sub-fields to that Repeater Field named faq_question
and faq_answers
.
In the Location section, choose the page you created for Questions and Answers.
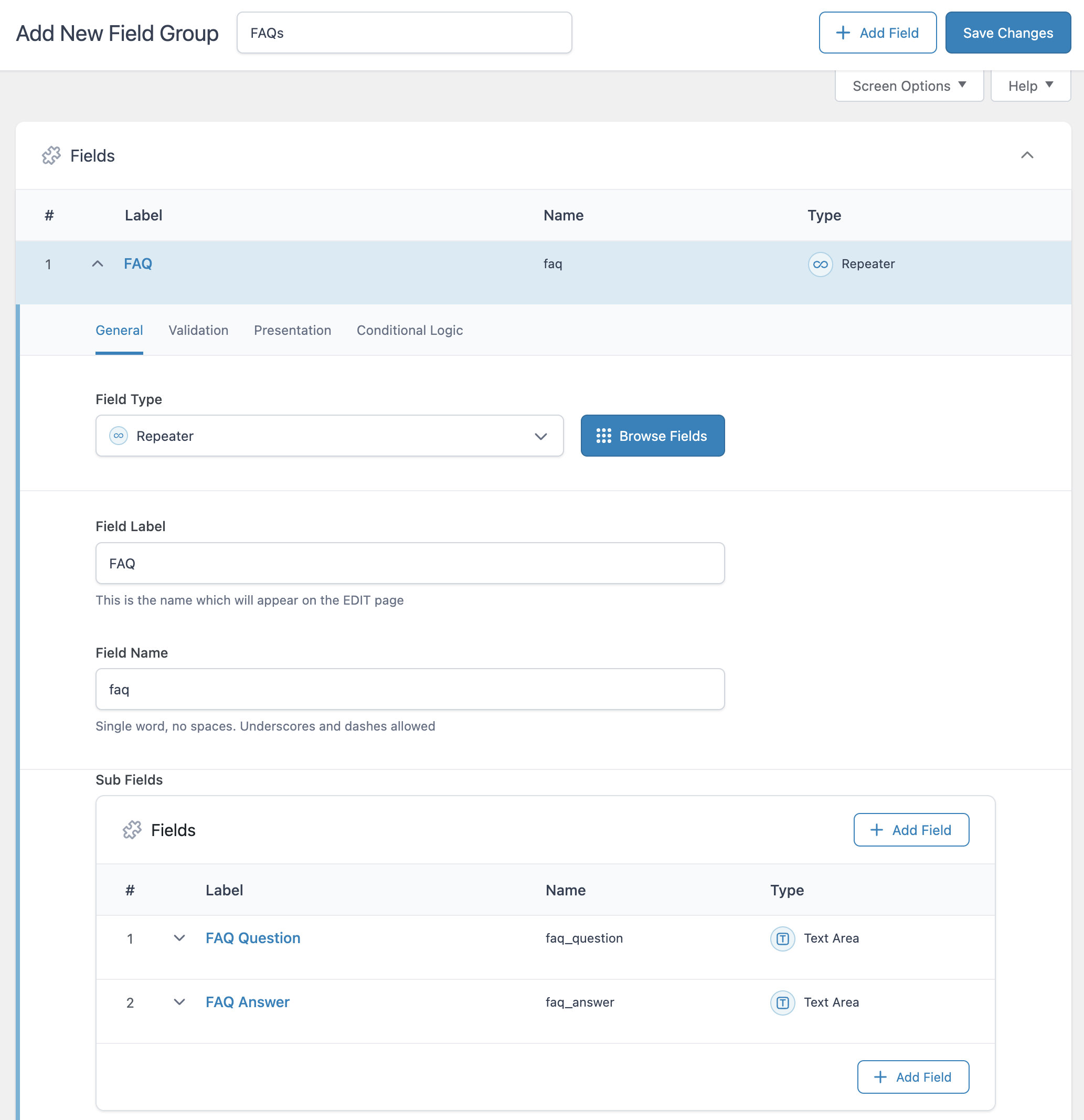
In the Location section, choose the page you created for Questions and Answers.
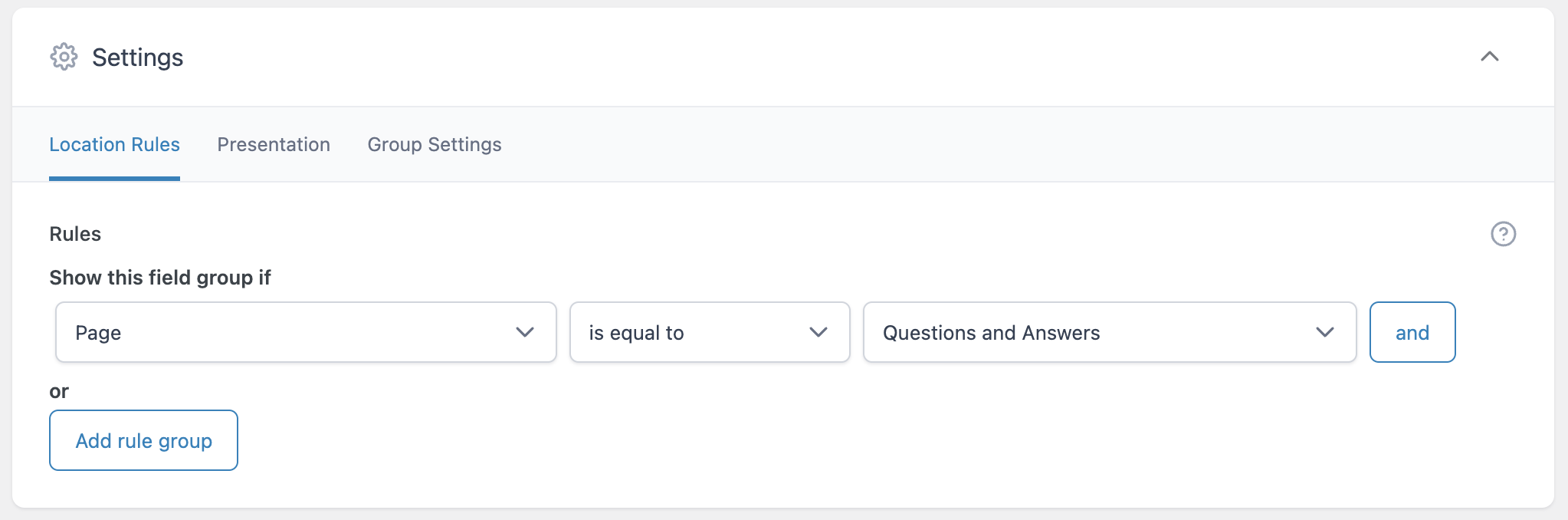
Creating a New Page Template
Now, create a new page template for the mentioned page. To do this, create a file named faq.php
in the root directory of your theme and add the following code:
<?php /* Template Name: Frequently Asked Questions */ ?>
You can, of course, edit the page template as you wish later. In our case, the only thing it will display is the page title and the questions and answers we are talking about in this post.
Now, add the following code. This code loops through all those questions and answers we will add to our page in the WordPress admin interface and displays them when the page is viewed. At the end, the faq.php
file will look like this:
<?php /* Template Name: Frequently Asked Questions */ ?>
<?php get_header(); ?>
<div id="primary" class="content-area">
<main id="main" class="site-main" role="main">
<?php
//My ACF Fields for reference
//faqs - field group
//faq_question - sub-field
//faq_answer - sub-field
// check if the repeater field has rows of data
if ( have_rows( 'faq' ) ):
?>
<div id="faq_container">
<?php
// loop through the rows of data
while ( have_rows( 'faq' ) ) : the_row();
?>
<div class="faq">
<div class="faq_question">
<span class="question"><?php echo get_sub_field( 'faq_question' ); ?></span>
<span class="accordion-button-icon fa fa-plus"></span>
</div>
<div class="faq_answer_container">
<div class="faq_answer"><?php echo get_sub_field( 'faq_answer' ); ?></div>
</div>
</div>
<?php
endwhile;
?>
</div>
<?php
endif;
?>
</main><!-- .site-main -->
</div><!-- .content-area -->
<?php get_footer(); ?>
We added additional HTML so that those questions and answers will be in a container, allowing manipulation with jQuery.
Assigning the Page Template We Created to the Questions and Answers Page
To assign the page template we created to the page we created earlier, go to the WordPress admin interface of that page and assign the new template to it.
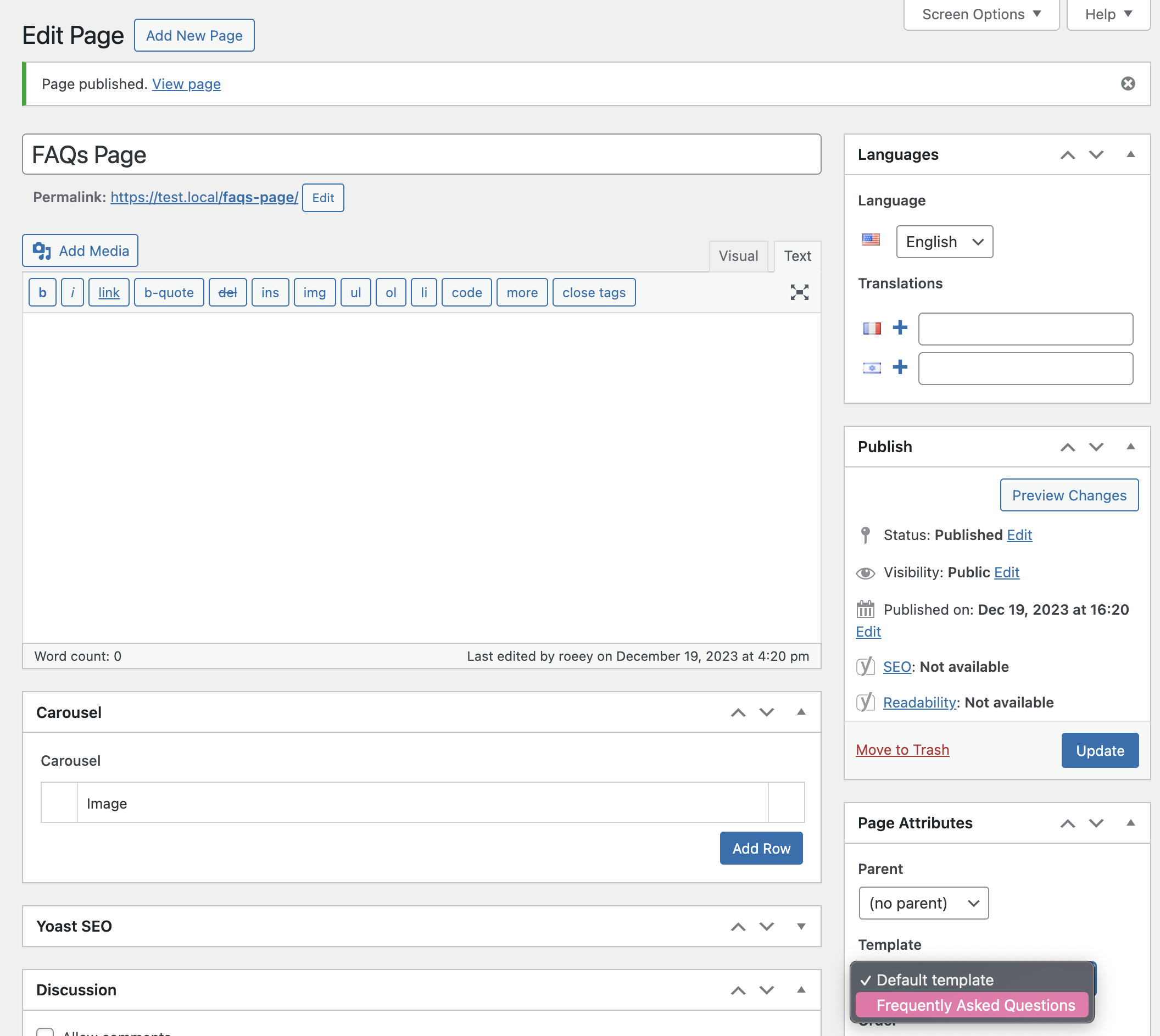
Adding jQuery & CSS
We will use jQuery to open and close the container upon clicking. Create a file named faq.js
in the js folder of your theme and add the following code to it:
jQuery(document).ready(function($) {
$('.faq_question').click(function() {
if ($(this).parent().is('.open')){
$(this).closest('.faq').find('.faq_answer_container').animate({'height':'0'},300);
$(this).closest('.faq').removeClass('open');
$(this).parent().find('.accordion-button-icon').removeClass('fa-minus').addClass('fa-plus');
}
else{
var newHeight =$(this).closest('.faq').find('.faq_answer').height() + 30 +'px';
$(this).closest('.faq').find('.faq_answer_container').animate({'height':newHeight},300);
$(this).closest('.faq').addClass('open');
$(this).parent().find('.accordion-button-icon').removeClass('fa-plus').addClass('fa-minus');
}
});
});
Create an additional file named faq.css
in the css folder of your theme and add the following code to it:
#faq_container {
border: 1px solid #e5e5e5;
margin-bottom: 10px;
padding: 0;
}
.faq {
border-bottom: 1px solid #eee;
}
.faq:last-child {
border: 0;
}
.faq_answer {
padding: 15px;
}
.faq_question {
margin: 0;
cursor: pointer;
font-weight: bold;
display: flex;
padding: 15px;
align-items: center;
transition: background .3s;
}
.faq_question:hover {
background: #e4e4fd;
}
.question {
margin-bottom: 5px;
display: table-cell;
width: 100%;
}
.faq_answer_container {
height: 0;
overflow: hidden;
}
.accordion-button-icon {
display: table-cell;
line-height: inherit;
opacity: .5;
filter: alpha(opacity=50);
vertical-align: middle;
}
Now let’s load these assets using enqueuing as you should already know, as mentioned in this guide. Add the following code to the functions.php
file, and please note that you should not copy the opening PHP tag:
<?php // DO NOT COPY THE OPENING PHP TAG
function faq_page()
{
if (is_page_template('faq.php')) {
wp_register_style('my-css', get_template_directory_uri() . '/css/faq.css', array(''), 1.01);
wp_enqueue_style('my-css');
wp_register_script('my-js', get_template_directory_uri() . '/js/faq.js', array('jquery'));
wp_enqueue_script('my-js');
wp_enqueue_style ( 'fontawesome' , '//maxcdn.bootstrapcdn.com/font-awesome/4.3.0/css/font-awesome.min.css', '' , '4.3.0', 'all' );
}
}
add_action('wp_enqueue_scripts', 'faq_page');
If you are dealing with a child theme, change the function
get_template_directory_uri()
toget_stylesheet_directory_uri()
.Also, note that we have added the font-awesome library, which we use for icons (plus and minus). However, it can definitely be done without it.
We’re done. Now, assuming you’ve added some questions and answers using the ACF repeater field on the mentioned page, if you preview the page, you should see something in the following style:
Feel free to ask questions and provide comments if something isn’t working in the comments below.
Good work, mate! Thank you.
Thanks )