This will be a short and concise post. If you’re not familiar with Slick Slider – it’s a JavaScript library that allows you to create sliders/carousels very conveniently. I wrote an article about using Slick Slider and you’re welcome to take a look if you’re not familiar.
In this post, we’ll see how to add various animations to Slick sliders using Animate.css. This is because Slick provides only two default types of animations for sliders: Fade & Slide.
So let’s say you’ve added a slider using the following HTML:
<div class="savvy-slick-carousel">
<div class="slick-img"><img src="IMAGE URL" alt="Slick Image 1" /></div>
<div class="slick-img"><img src="IMAGE URL" alt="Slick Image 2" /></div>
<div class="slick-img"><img src="IMAGE URL" alt="Slick Image 3" /></div>
<div class="slick-img"><img src="IMAGE URL" alt="Slick Image 4" /></div>
</div>
The JavaScript code to activate the slider looks like this:
$('.savvy-slick-carousel').slick({
dots: false,
infinite: true,
speed: 420,
autoplay: true,
autoplaySpeed: 4200,
slidesToShow: 1,
fade: true,
slidesToScroll: 1,
rtl: true
});
The result looks like this:
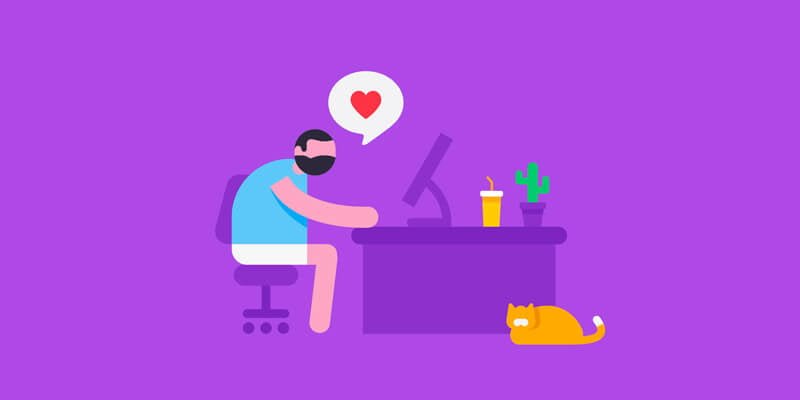
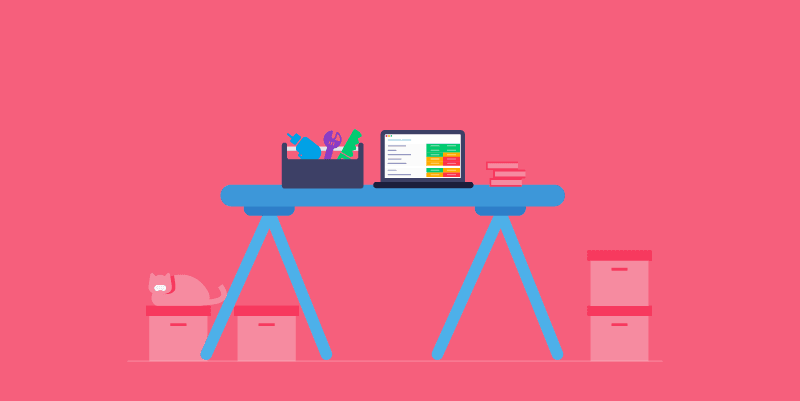
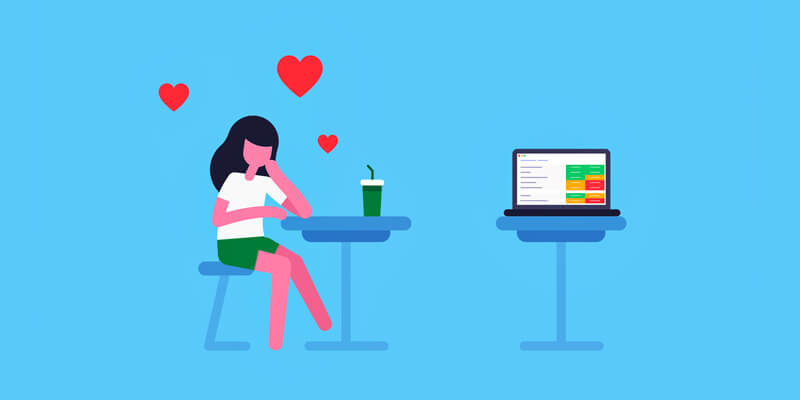
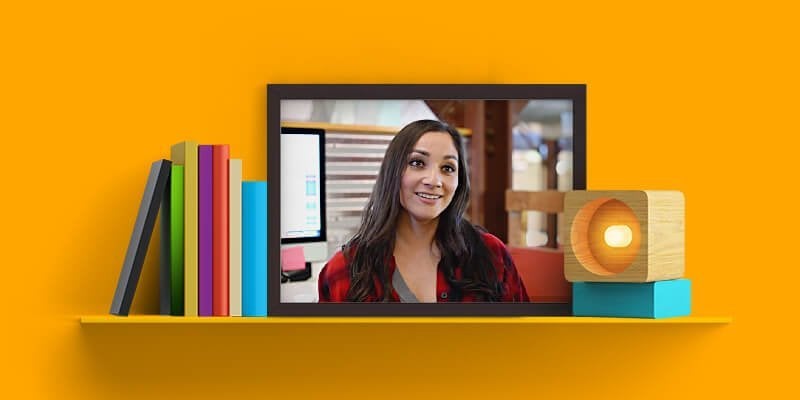
As you can see, this is a simple slider that displays one slide at a time and transitions using a basic Fade animation provided by the Slick Slider library.
Now, let’s see how to achieve the following result where images perform a ZoomOut and FadeOut on exit, and ZoomIn and FadeIn on entry, as shown in the example below:
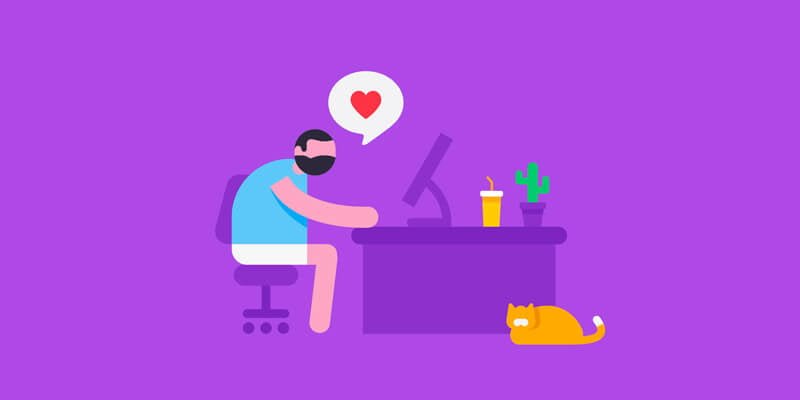
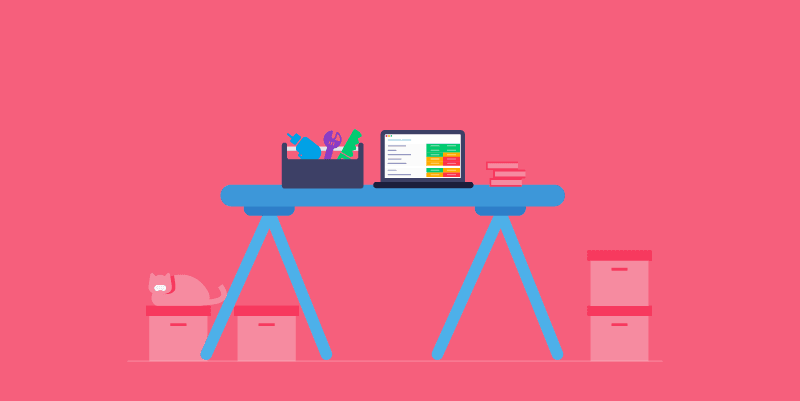
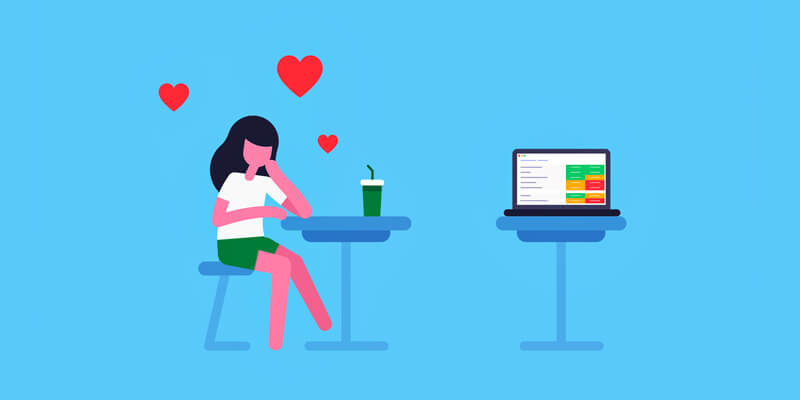
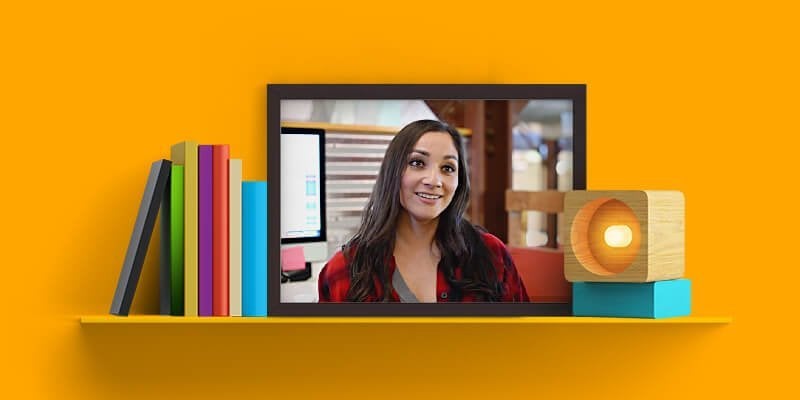
To achieve this, we’ll use the Animate.css library that we mentioned before. This library contains various CSS animations that can be applied to any element you desire.
Enqueue Animate.css in the standard way. If you’re unsure how to do this (similarly to enqueuing the Slick library), take a look at the Adding Assets (Js,Css) in WordPress Sites post.
Using Animate.css for Different Slider Animations
To apply an animation to a specific element using this library, all you need to do is add a class named animated
and one of the relevant Animate.css classes to the element, depending on the animation you want.
For Fade and Zoom animations, you can add the necessary classes using JavaScript precisely during the slide transition. The animated
class can be added directly in the HTML of the slider, as it remains constant and doesn’t change.
So, the HTML for the slider will look like this:
<div class="savvy-slick-carousel">
<div class="slick-img animated"><img src="IMAGE URL" alt="Slick Image 1" /></div>
<div class="slick-img animated"><img src="IMAGE URL" alt="Slick Image 2" /></div>
<div class="slick-img animated"><img src="IMAGE URL" alt="Slick Image 3" /></div>
<div class="slick-img animated"><img src="IMAGE URL" alt="Slick Image 4" /></div>
</div>
The slider activation will look like this:
var slides = $('.savvy-slick-carousel > div');
$('.savvy-slick-carousel').slick({
dots: false,
infinite: true,
speed: 0,
autoplay: true,
autoplaySpeed: 4200,
slidesToShow: 1,
fade: true,
slidesToScroll: 1,
rtl: true
}).on('beforeChange', function (event, slick, currentSlide, nextSlide) {
slides.removeClass('fadeInUp fadeOutUp zoomOut zoomIn');
slides.eq(currentSlide).addClass('fadeOutUp zoomOut');
slides.eq(nextSlide).addClass('fadeInUp zoomIn');
});
Note that you need to change the
speed
parameter to 0 as shown on line 6.
In this code, we’re selecting and storing the elements of each slide into a variable named slides
. Then, using the beforeChange
event of the Slick Slider, which triggers just before a slide changes, we remove the relevant classes from the current slide and add them to the next slide.
Additionally, by using the jQuery addClass
and removeClass
functions, we manage to remove the mentioned classes from the current slide and add the relevant ones to the next slide.
Note About Effects with Text Slides
In the case of images, you might not notice the issue with the basic Fade animation that Slick provides. However, if the slider were intended for text instead of images, you would see that during the animation, the text overlaps and becomes unclear. Pay attention:
In contrast, with the animation effects demonstrated with images, you can achieve a situation where text slides look better, avoiding a situation where text overlaps during transitions and looks unclear. Here’s how it looks after adding the animation:
And that’s it. If you enjoyed the post, feel free to check out the Creating Sliders with Vegas Slider post, which introduces an interesting slider library. Comments and ideas are welcome. 🙂