Delaying Javascript, in other words, Defer parsing of Javascript, is one of the messages you might receive when checking your website’s speed using tools like GTmetrix and Pingdom. But what does it actually mean to delay the parsing of Javascript?
So basically, this message indicates that the browser needs to process some content within a specific <script>
tag before it can continue loading the rest of the page. By delaying the processing of that script until it’s actually needed, we can improve the loading time of a page.
We won’t delve further into this, and in this post, we will focus exclusively on deferring Javascript for YouTube videos. If you’re interested in a more comprehensive explanation of the topic, take a look at the post How to Defer Javascript in WordPress Sites.
In one of the websites where I optimized speed and loading time, there was a YouTube video on the homepage. The page received a low score in speed testing tools, and I had to do something to address it.
The main issue was Defer Parsing of Javascript, as three of those problematic scripts were scripts from youtube.com. Here’s the note I received in GTmetrix:
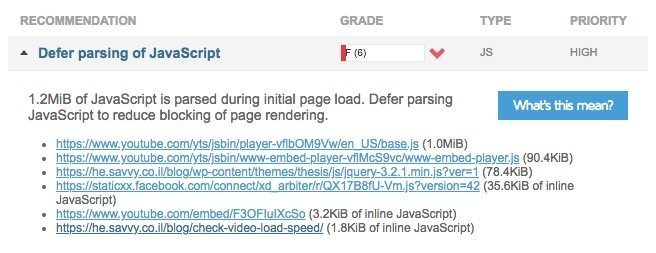
The scripts from youtube.com that you see in this image delayed the page load. In other words, only after these scripts were loaded (and note that these are heavy scripts), the rest of the page continued loading.
How to Delay Javascript for YouTube Videos?
Let’s explain how to solve this issue. When you embed a video in WordPress by copying the video’s URL from sites like YouTube or Vimeo, WordPress generates an <iframe>
for that video. It’s essentially the same <iframe>
you would get if you directly copied the embed code from YouTube.
Instead of using the URL to embed the video, go to YouTube and copy the embed code in the following way:
- Under the video, click on “Share“.
- Click on “Embed” that appears below it.
- Make any necessary changes in the embed options.
- Copy the embed code starting with “…iframe width>“.
Then, go to your website and paste the <iframe>
code where you want to display the video. The next step will be to slightly modify this code:
- Find the part …src=”https://www.youtube.com and change src to data-src.
- Add an empty parameter named “”=src. Ultimately, this will contain the URL from the data-src parameter that we just added.
Ultimately, your <iframe>
code will look like this:
<iframe width="560" height="315" src="" data-src="https://www.youtube.com/embed/123456789" frameborder="0" allowfullscreen></iframe>
Triggering Video Loading After Page Load Using Javascript
The <iframe>
we’ve created so far won’t load the video properly. Let’s add a bit of Javascript to load it only after the page has fully loaded.
function init() {
var vidDefer = document.getElementsByTagName('iframe');
for (var i = 0; i < vidDefer.length; i++) {
if (vidDefer[i].getAttribute('data-src')) {
vidDefer[i].setAttribute('src', vidDefer[i].getAttribute('data-src'));
}
}
}
window.onload = init;
This code simply copies the data-src
parameter to the src
parameter after the page has loaded. When this action takes place, the YouTube video will be displayed on your page. Notice the differences in GTmetrix results before and after applying this technique.
If you’re unsure how to add Javascript to your website, take a look at the post Adding Assets (Javascript and CSS Files) in WordPress.
Before Delaying Javascript for Videos:
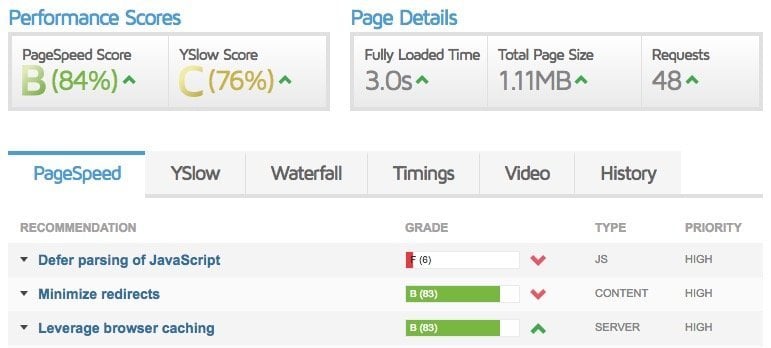
After Delaying Javascript for Videos:
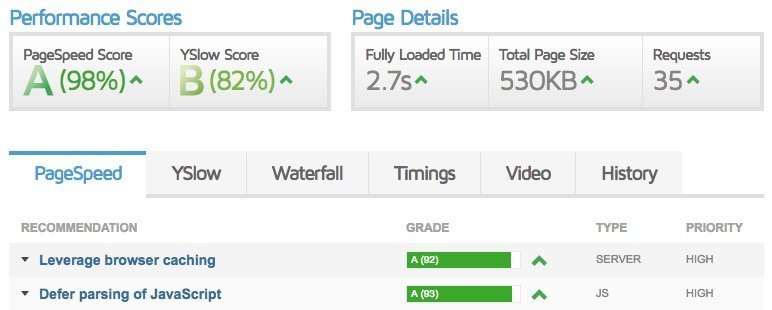
Notice that the score, server requests, and page size significantly improved. The score under Defer Parsing of Javascript jumped from F to A, and those notes we mentioned at the beginning of the post for this section disappeared.
Additional Considerations
In the case of the site where I optimized, the video was only present on the homepage, so I ensured that the Javascript code would only load on the homepage by adding a condition within the wp_enqueue_scripts
function.
It’s worth noting that this code will affect only <iframe>
elements with the data-src
parameter, so you don’t need to worry about it affecting other videos on your site that don’t have this parameter.
Another way to improve the loading time of embedded YouTube videos on your site can be found in the post Improving Loading Time of Embedded Videos with Lite YouTube. And if you find that the YouTube videos on your site aren’t responsive, check out the post explaining How to Make YouTube Videos Responsive in WordPress.
Thanks to Patrick Sexton for the JS Code.