Cookies are one of the most important web technology. Cookies were created to store persistent information about users in their browsers. A cookie is a piece of information from a specific website that is stored in the browser so that the same site can access and use the information stored in this cookie at a later stage.
When a specific user returns to a site, that same cookie provides information and allows the site to display specific settings or content for that user.
For example, cookies can store information such as items in a shopping cart, usernames and passwords for forms, and more. Advertisers use cookies to track the activity of users across different websites in order to display targeted advertisements.
Although this method is intended to provide users with a more personalized web experience, some view it as a privacy concern. This is why you see many websites displaying a message and requesting permission to use cookies. Usually, you’ll encounter these messages if you’re browsing from a European Union country, such as this example:
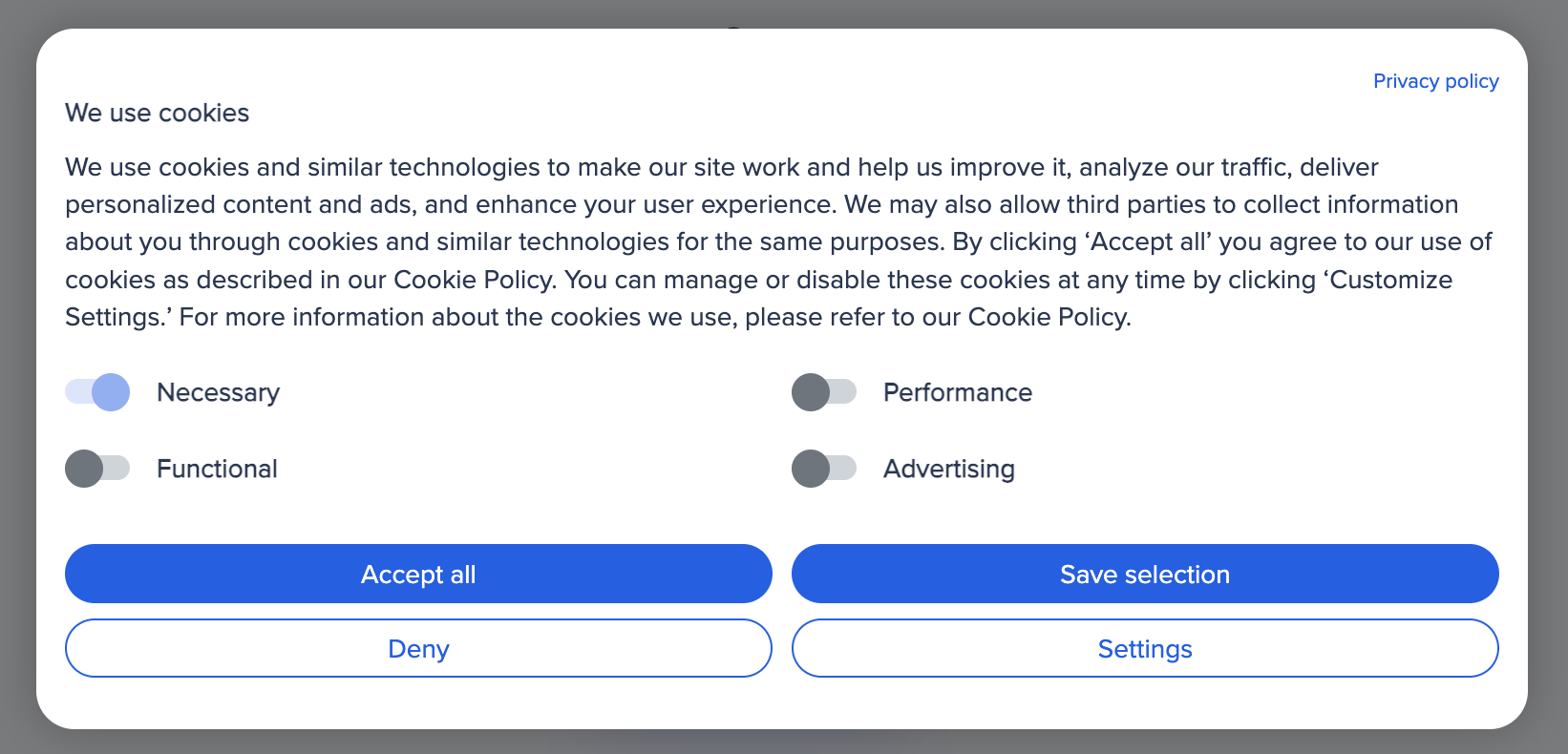
The Basics of Browser Cookies
When a browser requests a web page from a server, the cookies associated with that page are added to the request. This allows the server to receive the necessary information to remember information about users.
Cookies are essentially pieces of data that contain fields like name
and value
, along with several optional parameters. The values of these fields are strings in which you can store any text you want, according to the needs of your application or website. Cookies are stored as key & value pairs, for example:
username = John Doe
One common cookie, such as Google Analytics’ cookie, is named _ga
and is one of the most widely used cookies. It typically looks like this:
- Name: _ga
- Value: GA1.3.210706468.1583989741
- Domain: .example.com
- Path: /
- Expires / Max-Age: 2022-03-12T05:12:53.000Z
You can store up to 4096 bytes of data in a cookie, and there’s a limited number of cookies per domain. This number varies among different browsers.
A. Creating a Cookie with JavaScript
With JavaScript, you can create, read, and delete cookies using the document.cookie
property. For example, you can create a cookie like this:
document.cookie = "username=John Doe";
You can also add an expiration date to a cookie (in UTC time). By default, cookies are deleted when the browser is closed:
document.cookie = "username=John Doe; expires=Thu, 18 Dec 2013 12:00:00 UTC";
Using the path
parameter, you can specify to which path or page the cookie belongs. By default, a cookie belongs to the current page:
document.cookie = "username=John Doe; expires=Thu, 18 Dec 2013 12:00:00 UTC; path=/";
B. Reading Cookies with JavaScript
You can read cookies using JavaScript like this:
let x = document.cookie;
Using document.cookie
will return all cookies as a string: cookie1=value; cookie2=value; cookie3=value;
C. Modifying Cookies with JavaScript
You can modify cookies using JavaScript similarly to how you create them. In this case, the old cookie will be overwritten by the new one you create:
document.cookie = "username=John Smith; expires=Thu, 18 Dec 2013 12:00:00 UTC; path=/";
D. Deleting Cookies with JavaScript
Deleting a cookie is straightforward—you don’t need to provide the cookie’s value. Just set the expire
parameter to a date that has already passed:
document.cookie = "username=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/;";
Make sure to specify the correct path to ensure you’re deleting the right cookie. Certain browsers won’t allow you to delete a cookie if you don’t specify the path.
E. Cookie String Representation
The document.cookie
property looks like a regular text string, but it’s not. Even if you write the entire cookie string to document.cookie
, when you read it, you’ll only get the name-value pairs.
If you set a new cookie, old cookies won’t be deleted. The new cookie will be appended to document.cookie
, so if you read document.cookie
again, you’ll get something like:
cookie1 = value; cookie2 = value;
For the purpose of understanding cookie string representation, use the buttons below to create, display, and delete cookies.
If you want to find the value of a specific cookie, you need to create a JavaScript function that searches for the value within its string.
Example – JavaScript Cookie
In the following example, we’ll create a cookie that stores the visitor’s name on the website. The first time the visitor arrives on the page, they will be prompted to enter their name, and that name will be stored in a cookie. The next time the user visits the page, they will receive a “Welcome Roee” message.
For the example, we will create three JavaScript functions:
- A function to set the value of the cookie.
- A function to read the value of the cookie.
- A function to check the value of the cookie.
These functions and the code below were taken and adapted from this source.
1. Function to Set a Cookie
First, we’ll create a function that sets the user’s name in a cookie:
function setCookie(cname, cvalue, exdays) {
const d = new Date();
d.setTime(d.getTime() + (exdays*24*60*60*1000));
let expires = "expires="+ d.toUTCString();
document.cookie = cname + "=" + cvalue + ";" + expires + ";path=/";
}
The parameters of this function are the cookie name (cname), the cookie value (cvalue), and the number of days until the cookie expires (exdays).
The function sets the cookie by concatenating the cookie name, value, and the expiration text.
2. Function to Read a Cookie
Now, we’ll create a function that returns the value of a specific cookie:
function getCookie(cname) {
let name = cname + "=";
let decodedCookie = decodeURIComponent(document.cookie);
let ca = decodedCookie.split(';');
for(let i = 0; i <ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') {
c = c.substring(1);
}
if (c.indexOf(name) == 0) {
return c.substring(name.length, c.length);
}
}
return "";
}
We won’t explain how this function works here, feel free to ask in the comments…
3. Function to Check a Cookie
The final function checks if the cookie is defined. If it is, it displays a welcome message; if not, it prompts the user to enter their name and stores it in a cookie for 365 days using the setCookie
function we defined earlier:
function checkCookie() {
let username = getCookie("username");
if (username != "") {
alert("Welcome again " + username);
} else {
username = prompt("Please enter your name:", "");
if (username != "" && username != null) {
setCookie("username", username, 365);
}
}
}
And that’s it! Before we conclude, note that there are libraries that simplify working with cookies. In any case, for further reading and inspiration, check out these resources:
Thanks, Very Helpful & Nice Blog!