From time to time, I encounter a situation where I need to know the ID of a post or page in WordPress, whether for shortcodes, template building, or any other purpose. I’ve found myself needing to find the ID of a page numerous times while working with WordPress over the years.
Unfortunately, WordPress does not allow by default to display these IDs conveniently in the WordPress admin panel.
One way to discover these IDs is by visiting the edit page of that specific page/post and checking its URL. It will look something like:
https://savvy.co.il/wp-admin/post.php?post=XXXX&action=edit
In this example, XXXX is the post ID – the unique identifier in WordPress’s database. However, this method is cumbersome and time-consuming. There should be an easier way to display these IDs, and fortunately, there is…
Displaying Post IDs Using Code
If you want to display post IDs yourself and in a convenient way, take a look at the code below. This code should be placed in the functions.php
file of your plugin or theme, but before you start, remember that you need to use a child theme or create one if it doesn’t exist. Check out this guide on how to do it.
Adding Columns to WordPress Admin Dashboard
WordPress provides excellent tools to modify the list of posts in the admin dashboard, allowing for the easy creation of new columns.
We need to use filters to add columns and actions to add content. Here’s an example of how to modify the list of posts in the admin dashboard:
add_filter( 'manage_posts_columns', 'sv_add_id_column', 5 );
add_action( 'manage_posts_custom_column', 'sv_id_column_content', 5, 2 );
function sv_add_id_column( $columns ) {
$columns['sv_id'] = 'ID';
return $columns;
}
function sv_id_column_content( $column, $id ) {
if( 'sv_id' == $column ) {
echo $id;
}
}
This is essentially all we need. These hooks allow us to add a column by modifying the columns array. The key of the array is the column identifier, and its value is what will be displayed in the WordPress admin dashboard under that column name.
Notice that the sv_custom_columns_content
function takes two parameters, the column name and the ID of the displayed post. All that remains is to simply print this ID in the column we created. Here’s an image of the result as it appears in the WordPress admin dashboard:
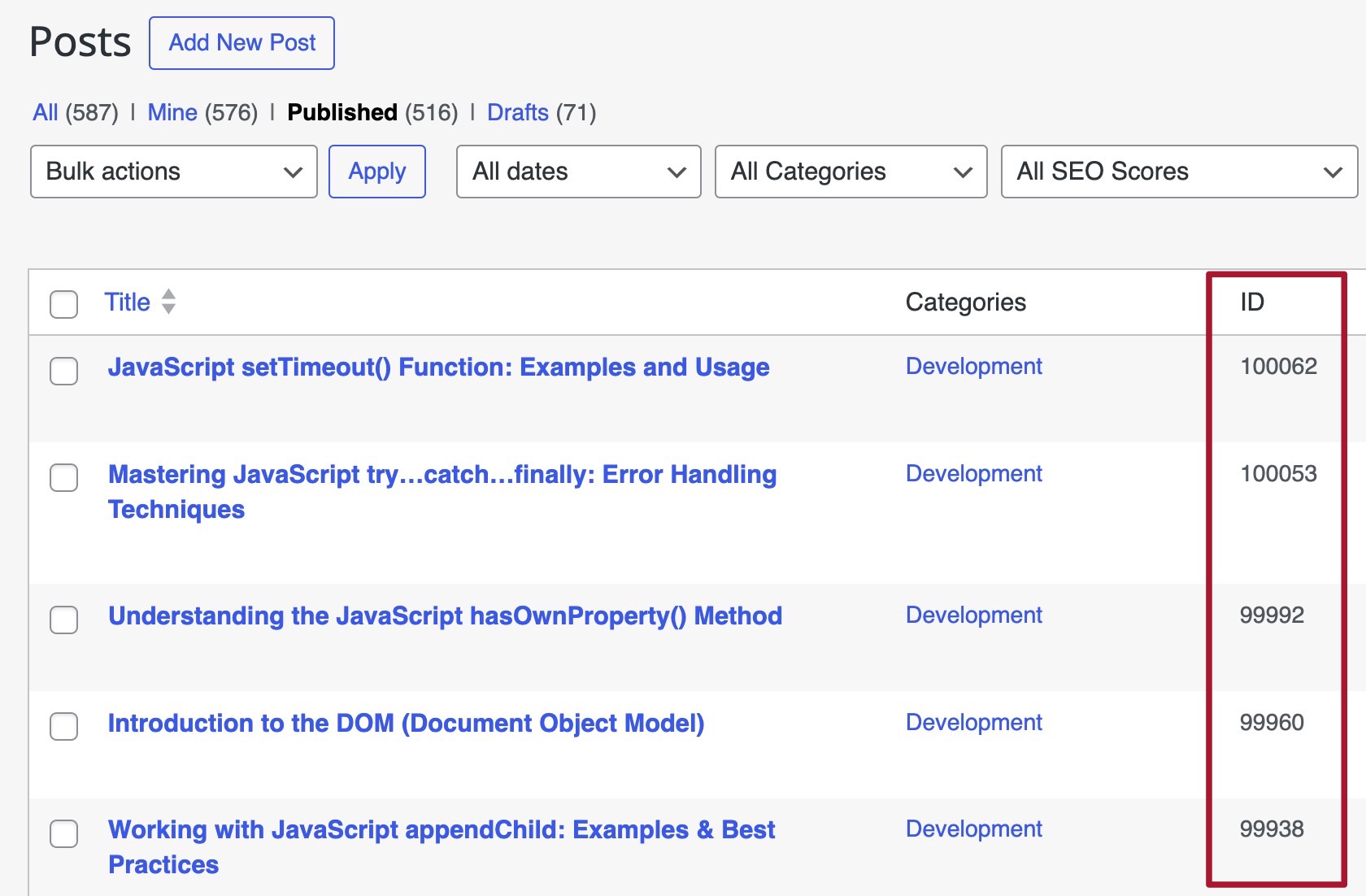
Finding the Right Hooks
These two functions above are all we need. The second part of the puzzle is where to apply the hook. The hooks we used target only regular posts, and the ID column will not appear for pages or any other content type.
These hooks are called “variable hooks” because they belong to a set of hooks and are written as follows:
manage_[post_type_or_element]_columns
manage_[post_type_or_element]_custom_column
Based on this, we can work with posts, pages, and custom content types. To display the ID column for posts, pages, comments, media, and even users, we need to use the following code:
function sv_add_id_column( $columns ) {
$checkbox = array_slice( $columns , 0, 1 );
$columns = array_slice( $columns , 1 );
$id['sv_id'] = 'ID';
$columns = array_merge( $checkbox, $id, $columns );
return $columns;
}
function sv_id_column_content( $column, $id ) {
if( 'sv_id' == $column ) {
echo $id;
}
}
// posts & posts types //
add_filter( 'manage_posts_columns', 'sv_add_id_column', 5 );
add_action( 'manage_posts_custom_column', 'sv_id_column_content', 5, 2 );
// pages //
add_filter( 'manage_pages_columns', 'sv_add_id_column', 5 );
add_action( 'manage_pages_custom_column', 'sv_id_column_content', 5, 2 );
// users //
add_action( 'manage_users_columns', 'sv_add_id_column', 5 );
add_filter( 'manage_users_custom_column', 'sv_id_column_content' , 5, 2 );
// media
add_filter( 'manage_media_columns','sv_add_id_column', 5 );
add_action( 'manage_media_custom_column', 'sv_id_column_content', 5, 2 );
// comments //
add_action( 'manage_edit-comments_columns', 'sv_add_id_column', 5 );
add_action( 'manage_comments_custom_column', 'sv_id_column_content', 5, 2 );
In order to add the ID column for all taxonomies – categories, tags, etc., we use a foreach loop:
// all taxonomies //
function sv_id_taxonomy_columns( $columns ) {
$columns['my_term_id'] = 'ID';
return $columns;
}
function sv_id_taxonomy_columns_content( $content, $column_name, $term_id ) {
if ( 'my_term_id' == $column_name ) {
$content = $term_id;
}
return $content;
}
$taxonomies = get_taxonomies();
foreach ( $taxonomies as $taxonomy ) {
add_action( 'manage_edit-' . $taxonomy . '_columns', 'sv_id_taxonomy_columns');
add_filter( 'manage_' . $taxonomy . '_custom_column', 'sv_id_taxonomy_columns_content', 10, 3 );
}
Displaying the ID in the Second Column
Currently, the ID column appears somewhere in the last column. But what if we wanted to display the ID after the first column column for convenience (after the checkbox column)?
Theoretically, we could merge the columns array with our new array, but since the first column is the checkbox (the first element in the original array) it’s a bit tricky, and that’s because we want the ID column to appear after the checkbox column. So we’ll still merge the arrays, but before we do that, we are going to split the arrays.
The first array will contain the checkbox (the first element in the original array) and the second array will contain the rest of the elements. We merge the array containing the checkbox with the array containing our ID, and then with the array containing the other elements.
A look at the code should clarify the operation better, and the result is shown in the image below:
add_filter( 'manage_posts_columns', 'sv_add_id_column', 5 );
add_action( 'manage_posts_custom_column', 'sv_id_column_content', 5, 2 );
function sv_add_id_column( $columns ) {
$checkbox = array_slice( $columns , 0, 1 );
$columns = array_slice( $columns , 1 );
$new['sv_id'] = 'ID';
$columns = array_merge( $checkbox, $new, $columns );
return $columns;
}
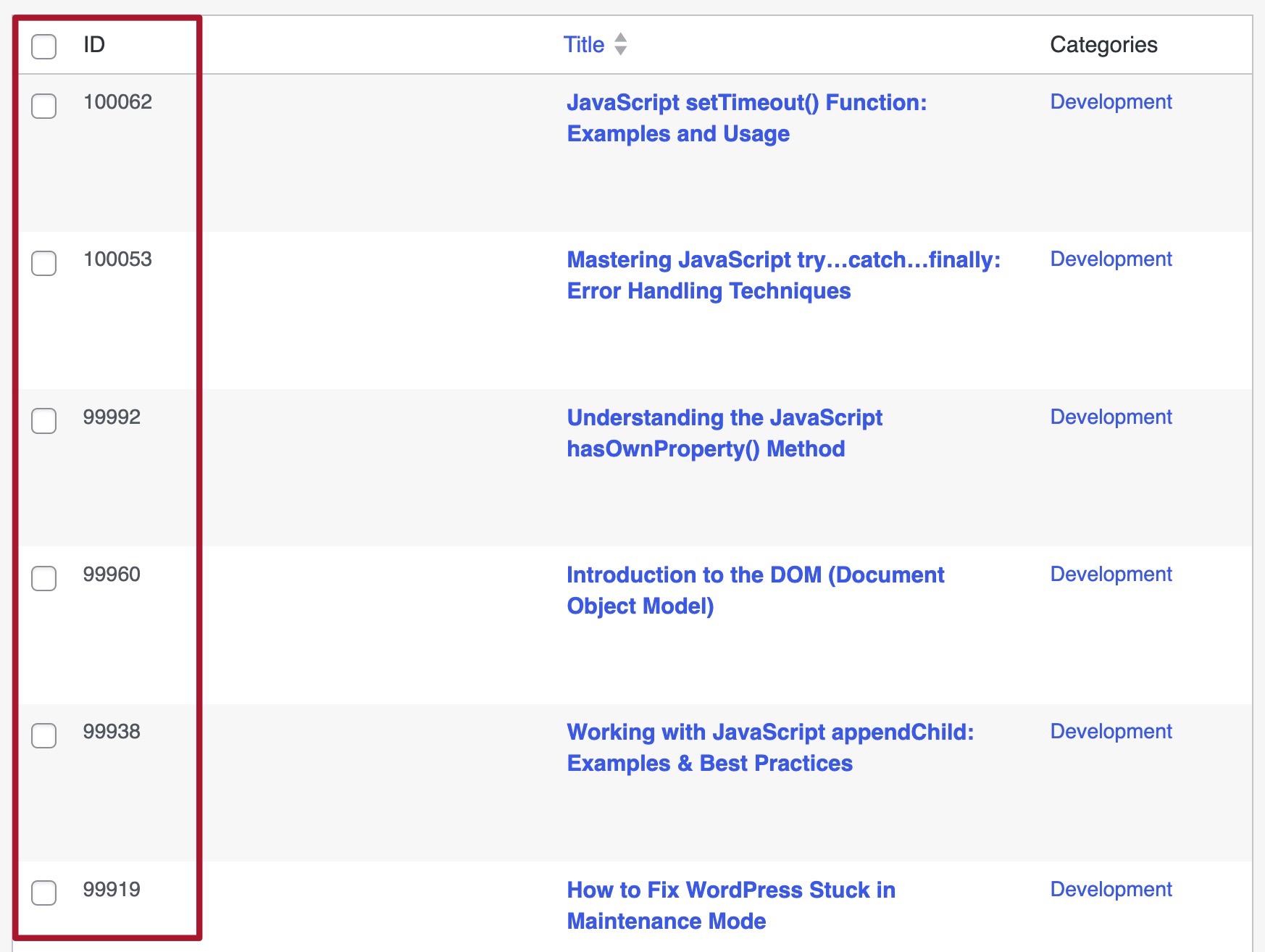
Conclusion
This example perfectly reflects the modularity of WordPress. By the way, in older versions of WordPress (2.5 and below), the ID was displayed by default, but since it was not relevant to most users, it was decided to remove it. And from the moment it was removed, all sorts of plugins began to emerge displaying the ID such as the Reveal IDs plugin.
Want to make the admin panel even more visual? You can also add a featured image column to the post list using a simple code snippet — no plugin required.