Optimizing your website’s performance involves many aspects, and one critical area is managing the Document Object Model (DOM) size. A lean, efficient DOM enhances rendering speed, reduces memory usage, and improves overall user experience.
If Google PageSpeed Insights flags your site with the warning “Avoids an excessive DOM size,” this post will help you understand why this matters and how to fix it.
What Is DOM Size?
The DOM (Document Object Model) is a hierarchical representation of your webpage’s structure. It serves as an interface between your HTML and the browser, allowing scripts to dynamically update content, style, and structure.
Every element, attribute, and text node in your HTML contributes to the DOM tree, creating a structure the browser must parse, render, and maintain.
The larger the DOM, the harder the browser works. This includes:
- Parsing: Reading and interpreting the HTML to build the DOM tree.
- Rendering: Combining the DOM with CSS to create the Render Tree, which dictates the page’s visual layout.
- Layout and Painting: Calculating element positions and painting them on the screen.
- Reflows and Repaints: Changes to the DOM or CSS trigger reflows (layout recalculations) and repaints (visual updates), which are more resource-intensive with a large DOM.
A large DOM also hinders JavaScript performance. Frameworks like React, Angular, or Vue must traverse and manipulate the DOM, slowing down as the node count increases.
For mobile users, the impact is even greater. Devices with limited memory and processing power may struggle with large DOMs, causing slow loads, laggy interactions, and potential browser crashes.
“A smaller DOM isn’t just about performance; it’s about creating a seamless and intuitive user experience.”
Additionally, a smaller, well-organized DOM improves maintainability. Simplified HTML and reduced nesting make debugging and updates easier. It also boosts accessibility, helping screen readers interpret content more effectively.
Why Is a Large DOM Problematic?
Having an excessively large DOM can lead to several performance issues:
- Slower Rendering: A larger DOM takes more time for the browser to process and render.
- Laggy User Interactions: Heavy DOM structures can delay responses to user inputs like clicks and scrolls.
- Increased Memory Usage: More nodes require more memory, which can overwhelm devices with limited resources, especially mobile devices.
How to Identify DOM Size Issues
To identify whether your site has an oversized DOM, use tools like Google PageSpeed Insights or Chrome DevTools:
<!-- Using Chrome DevTools -->
1. Open DevTools (Right-click on the page and select 'Inspect' or press Ctrl+Shift+I).
2. Navigate to the 'Elements' tab to explore the DOM tree.
3. Use the 'Performance' tab to record and analyze page performance, paying attention to DOM-related metrics.
Here’s how the warning looks in Google PageSpeed Insights:
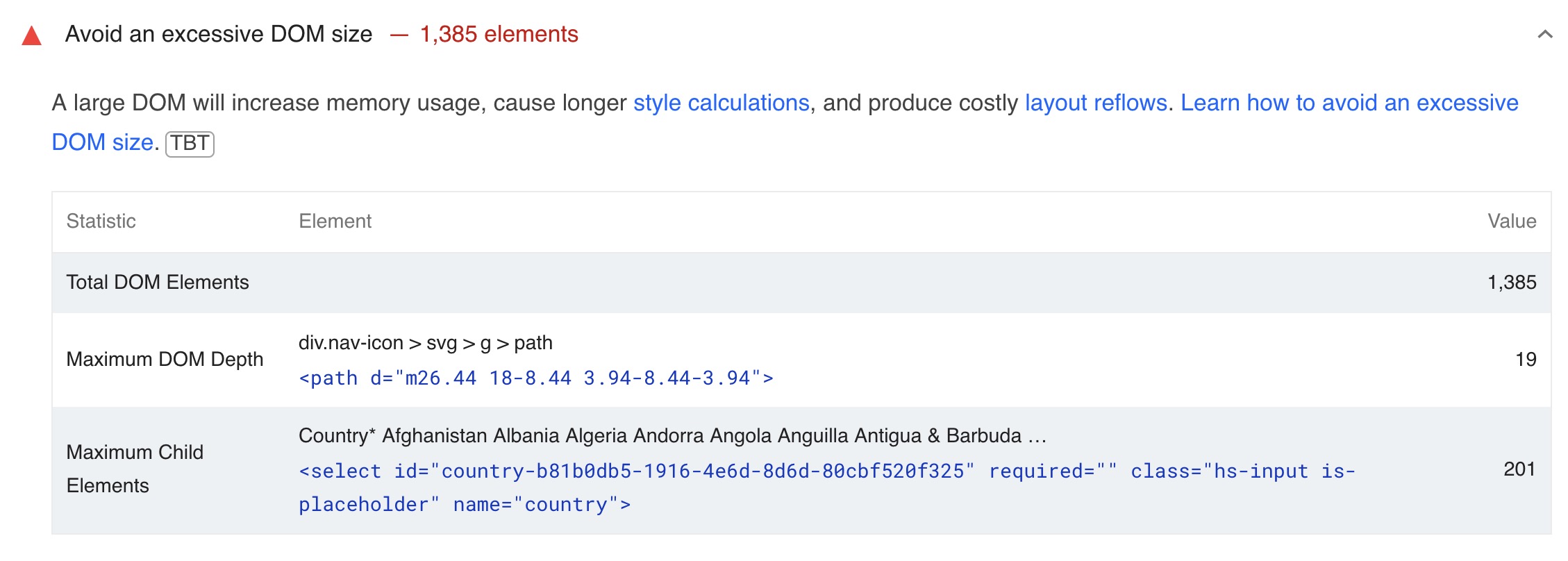
Since many use Elementor, be aware that using this plugin comes with a performance cost, particularly regarding the DOM & the DOM size and complexity.
Strategies for Reducing DOM Size
Here are actionable strategies to reduce your DOM size:
- Simplify Your HTML: Replace unnecessary divs and deeply nested elements with semantic HTML.
- Optimize CSS: Minimize the use of rules that require the browser to generate additional elements, like
:before
and:after
. - JavaScript Optimization: Use efficient methods to manipulate the DOM dynamically, avoiding excessive node creation.
Example 1: Simplifying HTML Structure
<!-- Unoptimized HTML -->
<div class="wrapper">
<div class="header"><h1>Page Title</h1></div>
<div class="content">
<div class="text"><p>Paragraph text...</p></div>
</div>
</div>
<div class="footer"><p>Footer content</p></div>
<!-- Optimized HTML -->
<main>
<header><h1>Page Title</h1></header>
<article>
<p>Paragraph text...</p>
</article>
<footer><p>Footer content</p></footer>
</main>
Explanation: The optimized version uses semantic HTML tags like <main>
, <article>
, and <footer>
, reducing the number of divs and simplifying the DOM structure.
“Switching to semantic HTML not only streamlines your DOM but also improves accessibility for users relying on assistive technologies.”
Example 2: Optimized vs. Unoptimized CSS
<!-- HTML Structure -->
<div class="container">
<h2>Main Title</h2>
<p>First paragraph.</p>
<p>Second paragraph.</p>
</div>
/* Unoptimized CSS */
.container p {
margin-top: 20px;
margin-bottom: 20px;
}
/* Optimized CSS */
.container > * + * {
margin-top: 20px;
}
Explanation: The unoptimized CSS applies margins to all paragraphs, leading to unnecessary spacing at the top and bottom.
The optimized version uses the adjacent sibling selector (> * + *
) to apply margins only between elements, resulting in cleaner and more efficient styling.
Example 3: Dynamic Content with JavaScript
// Unoptimized JavaScript
const container = document.getElementById('dynamic-content');
const title = document.createElement('h2');
title.textContent = 'Dynamic Title';
container.appendChild(title);
const paragraph = document.createElement('p');
paragraph.textContent = 'Dynamic text...';
container.appendChild(paragraph);
// Optimized JavaScript
document.getElementById('dynamic-content').innerHTML = '<h2>Dynamic Title</h2><p>Dynamic text...</p>';
Explanation: The optimized JavaScript reduces multiple DOM operations by directly assigning the HTML content, improving the performance of dynamic content updates.
Conclusion
Avoiding an excessive DOM size is a common performance issue that often goes unnoticed by inexperienced developers, who may struggle to address it effectively without proper guidance.
Optimizing your DOM size is essential for delivering a fast, seamless user experience. By simplifying your HTML, optimizing your CSS, and using efficient JavaScript practices, you can ensure your site runs smoothly, even on resource-constrained devices.