A function is a set of declarations (statements in English) that take input, perform a certain calculation, and provide output. In other words, a function is a set of declarations that perform a number of actions or calculations and then return the result to the user.
The idea is to encapsulate a number of repetitive actions and create a function from them, so instead of writing the same code and the same actions over and over again for different inputs, you can simply call the same function and save rewriting the code each time.
Just like in any programming language, JavaScript also supports functions, and you’ve probably used such functions and others in JavaScript, such as the built-in function alert()
.
However, JavaScript allows you to create your own defined functions. You can create functions in JavaScript using the function
keyword. The basic syntax for creating a function looks like this:
function functionName(Parameter1, Parameter2, ..)
{
// Function body
}
As you can see, to create a function in JavaScript, you need to start with the function
keyword, then write the function name, and then the parameters it receives in parentheses. The part inside the curly braces is the body of the function itself.
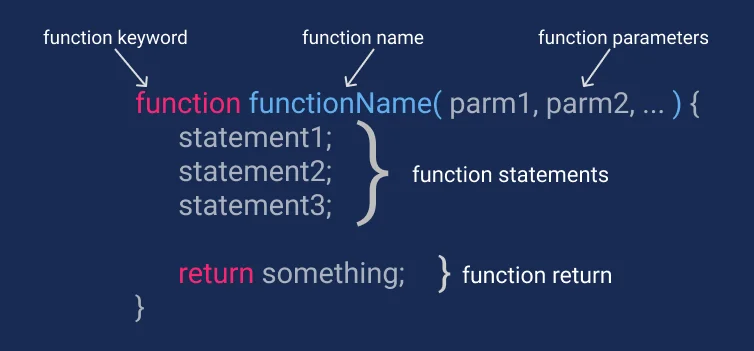
Function Definition
Let’s see some rules for creating a function in JavaScript. First of all, every function must start with the keyword function
, followed by:
- The name of the function chosen by the developer. This name should be unique.
- A list of parameters inside parentheses and separated by commas.
- A list of declarations or actions in the function body, enclosed in curly braces.
Here’s an example:
function calcAddition(number1, number2)
{
return number1 + number2;
}
In this example, we created a function called calcAddition
. The function takes two numbers as parameters and returns the sum of the numbers as a result using the return statement (which we’ll talk about later).
Function Parameters
Let’s talk a bit about the parameters of JavaScript functions. These parameters are the information passed to the function. In the example we provided above, the role of the calcAddition
function is to calculate the sum of two numbers. As explained, those numbers are passed to the function within parentheses after the function name, separated by commas.
Terminology-wise, the parameters of a function in JavaScript are the names you wrote inside the parentheses when defining the function. The arguments are the actual values you pass to the function.
A JavaScript function can accept up to 255 parameters, but it’s reasonable to assume that you’ll rarely need such a large number. Also, note that there’s no need to define the variable type (Integer, String, etc.) of those parameters in JavaScript.
Calling a JavaScript Function
After you’ve defined the function, the next step is to call that function (to use it). You can call a function by writing its name along with any values as the number of parameters the function accepts (inside parentheses) and ending with a semicolon.
functionName( Value1, Value2, ..);
Here’s a code example that demonstrates working with functions in JavaScript:
<script type = "text/javascript">
// Function definition
function welcomeMsg(name) {
document.write("Hello " + name + " welcome to Colombia");
}
// Creating a variable
var nameVal = "Admin";
// Calling the function
welcomeMsg(nameVal);
</script>
The result will be:
Hello Admin welcome to Colombia
Return Statement – The Value a Function Returns
There are situations where we want the function to return specific values after performing certain actions. In such cases, we can use the return statement. The return statement that the function can return is optional and usually appears as the last line of the function’s body.
Take another look at the first example of the calcAddition
function. The function calculates the sum of the two numbers and returns the result using the same return statement. The basic syntax of the return statement looks like this:
return value;
The return statement starts with the keyword return, followed by the value you want to return. You can also use any expression when returning the value, like in this example:
return value + 1;
Up to this point. If you found interest in this post, you might want to take a look at the post Playing with JavaScript Arrays, or Introduction to Objects in JavaScript.
The post was inspired by this post and was translated with various additions. Good luck 🙂