Loops are a kind of ‘computer program’ that executes a set of commands or a specific piece of code multiple times until a certain condition is met. A loop eliminates the need to write the same set of commands multiple times. In other words, loops allow us to run a specific piece of code as many times as we want.
There are various types of loops, but in this post, we will specifically focus on the syntax of the for
loop in JavaScript and present several examples that use this type of loop.
Almost all high-level programming languages, including JavaScript of course, have
for
loops.
The for Loop in JavaScript
The for
loop performs iterations until the condition defined within it evaluates to false
. The syntax of the for
loop in JavaScript looks like this:
for ([initialExpression]; [condition]; [incrementExpression])
statement
If we refer to the same syntax in Hebrew, it might look something like this:
for ([מונה]; [תנאי]; [קצב התקדמות המונה])
statement
Here’s an explanation of the process that happens behind the scenes:
- The initialExpression (counter) is initialized.
- A check/calculation of the condition takes place. If the value received is
true
, the statement (i.e., the body of the loop) is executed, and the next iteration is carried out. If not, thefor
loop terminates. - The body of the loop (statement) is executed.
- If present, the incrementExpression is executed, essentially describing the counter’s rate of progression.
- We return to step 2 and check the condition again. This process repeats until the value in the condition evaluates to
false
.
It’s worth noting that the
initialExpression
can also declare a variable or any level of complexity.
I assume examples could explain much better than I can, so here are a few:
Example A
A simple example where we display a message 5 times using a for
loop.
Code:
// program to display text 5 times
const n = 5;
// looping from i = 1 to 5
for (let i = 1; i <= n; i++) {
console.log(`I love JavaScript.`);
}
Output:
I love JavaScript.
I love JavaScript.
I love JavaScript.
I love JavaScript.
I love JavaScript.
And here’s a table explaining how the loop works in this case:
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1
n = 5 | true | I love JavaScript. is printed.
i is increased to 2. |
2nd | i = 2
n = 5 | true | I love JavaScript. is printed.
i is increased to 3. |
3rd | i = 3
n = 5 | true | I love JavaScript. is printed.
i is increased to 4. |
4th | i = 4
n = 5 | true | I love JavaScript. is printed.
i is increased to 5. |
5th | i = 5
n = 5 | true | I love JavaScript. is printed.
i is increased to 6. |
6th | i = 6
n = 5 | false | The loop is terminated. |
As a side note and before the next example, here’s a flowchart of the
for
loop: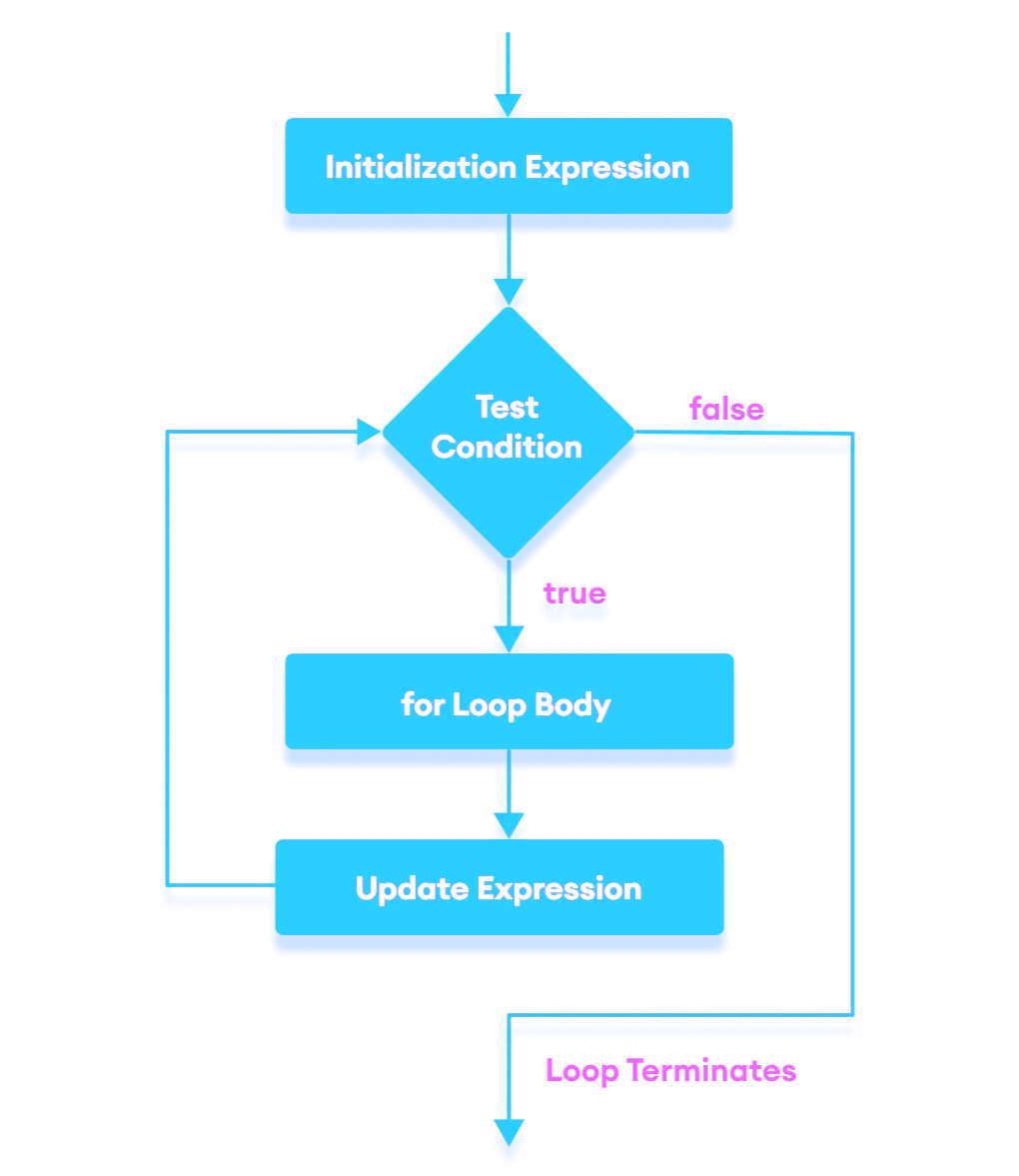
Example B
In this example, we’ll use a for
loop to display numbers from 1 to 5.
Code:
// program to display numbers from 1 to 5
const n = 5;
// looping from i = 1 to 5
// in each iteration, i is increased by 1
for (let i = 1; i <= n; i++) {
console.log(i); // printing the value of i
}
Output:
1
2
3
4
5
Here’s a table explaining how this loop works:
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1
n = 5 | true | 1 is printed.
i is increased to 2. |
2nd | i = 2
n = 5 | true | 2 is printed.
i is increased to 3. |
3rd | i = 3
n = 5 | true | 3 is printed.
i is increased to 4. |
4th | i = 4
n = 5 | true | 4 is printed.
i is increased to 5. |
5th | i = 5
n = 5 | true | 5 is printed.
i is increased to 6. |
6th | i = 6
n = 5 | false | The loop is terminated. |
Example C
In this example, we’ll use a for
loop to calculate the sum of all natural numbers from 1 to 100.
Code:
// program to display the sum of natural numbers
let sum = 0;
const n = 100
// looping from i = 1 to n
// in each iteration, i is increased by 1
for (let i = 1; i <= n; i++) {
sum += i; // sum = sum + i
}
console.log('sum:', sum);
Output:
sum: 5050
In this loop, the initial value of the sum
variable is 0
. The loop then iterates from i = 1 - 100
. In each iteration, the value of i
is added to sum
, and then the value of i
is increased by 1
.
When the value of i
reaches 101
, the condition fails, meaning it returns false
, and the loop terminates. The value of sum
will be equal to 0 + 1 + 2 + ... + 100
.
It’s worth mentioning that you could write this loop in a different way than the one presented above, as shown in the next example:
// program to display the sum of n natural numbers
let sum = 0;
const n = 100;
// looping from i = n to 1
// in each iteration, i is decreased by 1
for(let i = n; i >= 1; i-- ) {
// adding i to sum in each iteration
sum += i; // sum = sum + i
}
console.log('The sum of 1 to 10 is:',sum);
The result of this code will be the same as the result of the first example in this section. You probably know that in programming, you can achieve the same task in various ways. And while both of the approaches we presented are legitimate and correct, you should always consider how to write more readable and efficient code.
Example D
Since you’ll often work with arrays in JavaScript, let’s see how to use a for
loop to iterate over an array and display the even and odd numbers within it.
Code:
var numbers = [1, 4, 44, 64, 55, 24, 32, 55, 19, 17, 74, 22, 23];
var evenNumbers = [];
var oddNumbers = [];
for (var i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 != 1) {
evenNumbers.push(numbers[i]);
} else {
oddNumbers.push(numbers[i]);
}
}
console.log("The even numbers are: " + evenNumbers); // "The even numbers are: 4,44,64,24,32,74,22"
console.log("The odd numbers are: " + oddNumbers); // "The odd numbers are: 1,55,55,19,17,23"
Example E
Let’s look at a more complex example. In this example, we have a JavaScript function that contains a for
loop to count the number of options selected in an HTML select
element (which allows multiple selection).
The HTML looks like this:
<form name="selectForm">
<label for="musicTypes">Choose some music types, then click the button below:</label>
<select id="musicTypes" name="musicTypes" multiple>
<option selected>R&B</option>
<option>Jazz</option>
<option>Blues</option>
<option>New Age</option>
<option>Classical</option>
<option>Opera</option>
</select>
<button id="btn" type="button">How many are selected?</button>
</form>
And the JavaScript code looks like this:
function howMany(selectObject) {
let numberSelected = 0;
for (let i = 0; i < selectObject.options.length; i++) {
if (selectObject.options[i].selected) {
numberSelected++;
}
}
return numberSelected;
}
const btn = document.getElementById('btn');
btn.addEventListener('click', () => {
const musicTypes = document.selectForm.musicTypes;
console.log(`You have selected ${howMany(musicTypes)} option(s).`);
});
Note that in the for
loop inside the howMany
function, we declare and initialize the i
variable to 0
.
In the condition, we check if i
is less than the number of options in the select
element, and if so, we execute the following if
statement. Afterward, we increment the i
variable by 1
for each option selected in the select
element.
That’s all! For the sake of fairness, please note that the code examples were taken from various sources on the web. Feel free to ask any questions in the comments section of the post!