This guide will help you understand the use of the querySelector()
and querySelectorAll()
methods. We will learn how to easily find elements in the DOM using querySelector and querySelectorAll.
The term DOM refers to the document object model, which represents all HTML elements in a tree structure. Each element in that tree can be accessed through the API of modern browsers and using JavaScript.
In this post, I will present several tips and tricks for looping through any node (NodeList) in the DOM using the forEach()
loop.
JavaScript is a popular language due to its versatility and the API it provides in modern browsers.
Take a look at the following markup composed of various DOM elements that we will play with later:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>JavaScript DOM API querySelector and querySelectorAll Examples</title>
</head>
<body>
<div class="wrapper">
<div class="name">Liam</div>
<div class="name">Noah</div>
<div class="name">William</div>
<div class="name">James</div>
<div class="name">Benjamin</div>
<div class="name">Elijah</div>
</div>
<div id="country">USA</div>
<footer>Just some footer text</footer>
<script>
// code goes here
</script>
</body>
</html>
querySelectorAll – Example
Here is the syntax for querySelectorAll
:
listElements = parentNode.querySelectorAll(css-selector);
To search for elements in the DOM using querySelectorAll
, specifically in the case of the following code to find a div
with a class named name
in the HTML structure we presented earlier, add the following script somewhere at the bottom of the markup:
<script>
let name = document.querySelectorAll(".name");
console.log(name);
</script>
The function in line number 2 will return six elements in the DOM with the class name
.
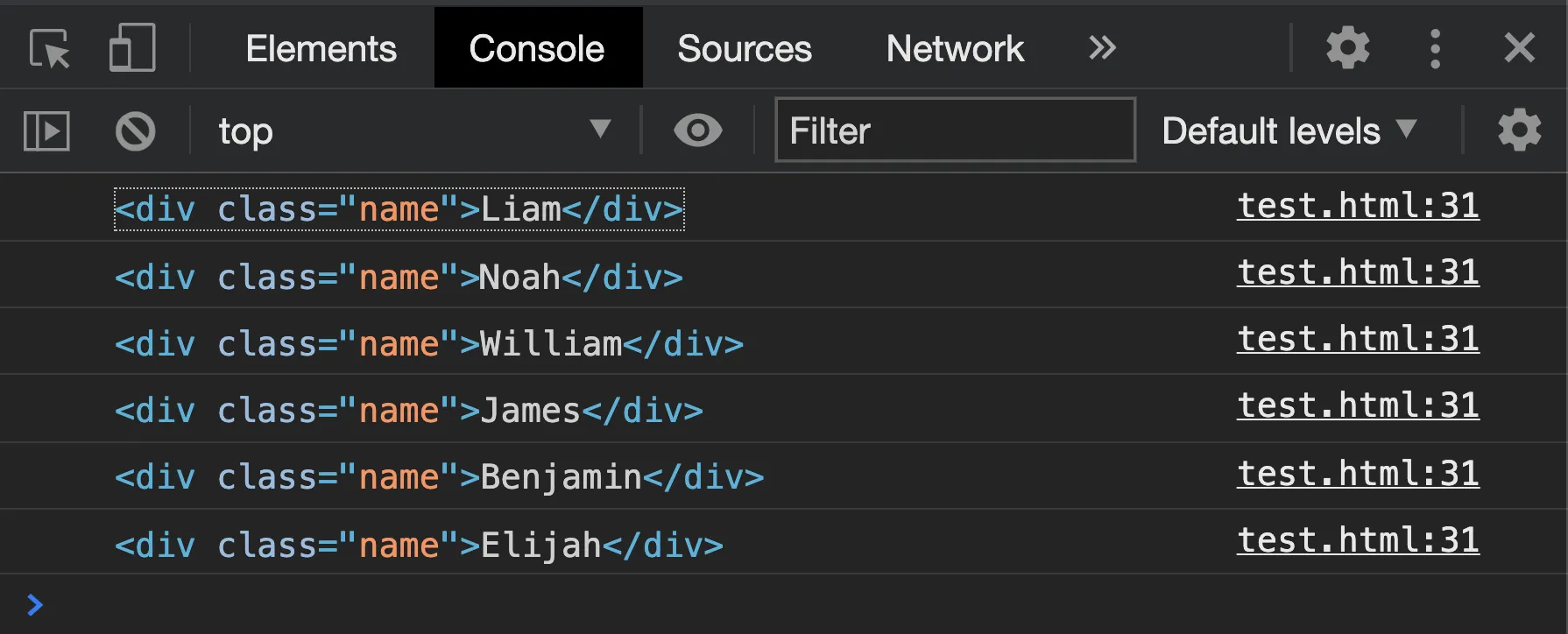
querySelector – Example
We’ve seen in the previous section how to perform a query on the DOM, but how do we traverse a single element in the DOM? In this case, we need to use querySelector
. Before we continue, take a look at the syntax for querySelector
:
domElement = parentNode.querySelector(css-selector);
The querySelector
method takes a CSS selector as an argument containing the ID or class and returns the requested element in the DOM.
<script>
let country = document.querySelector("#country");
console.log(country);
</script>
This function does exactly what we expect from it; it returned the element with the ID named country.

Looping through Elements in NodeList with querySelectorAll using forEach
Let’s see how to loop through all the elements in NodeList with querySelectorAll using the forEach loop.
Using the forEach loop on a NodeList is straightforward with querySelectorAll, but I’m somewhat skeptical about browser support in this case. Nonetheless, in the next section, we’ll see how to do it with support for all browsers.
This code will work for sure in Firefox and Chrome, but I’m not sure about Safari and Edge.
let name = document.querySelectorAll(".name");
name.forEach((elements) => {
console.log(elements);
})
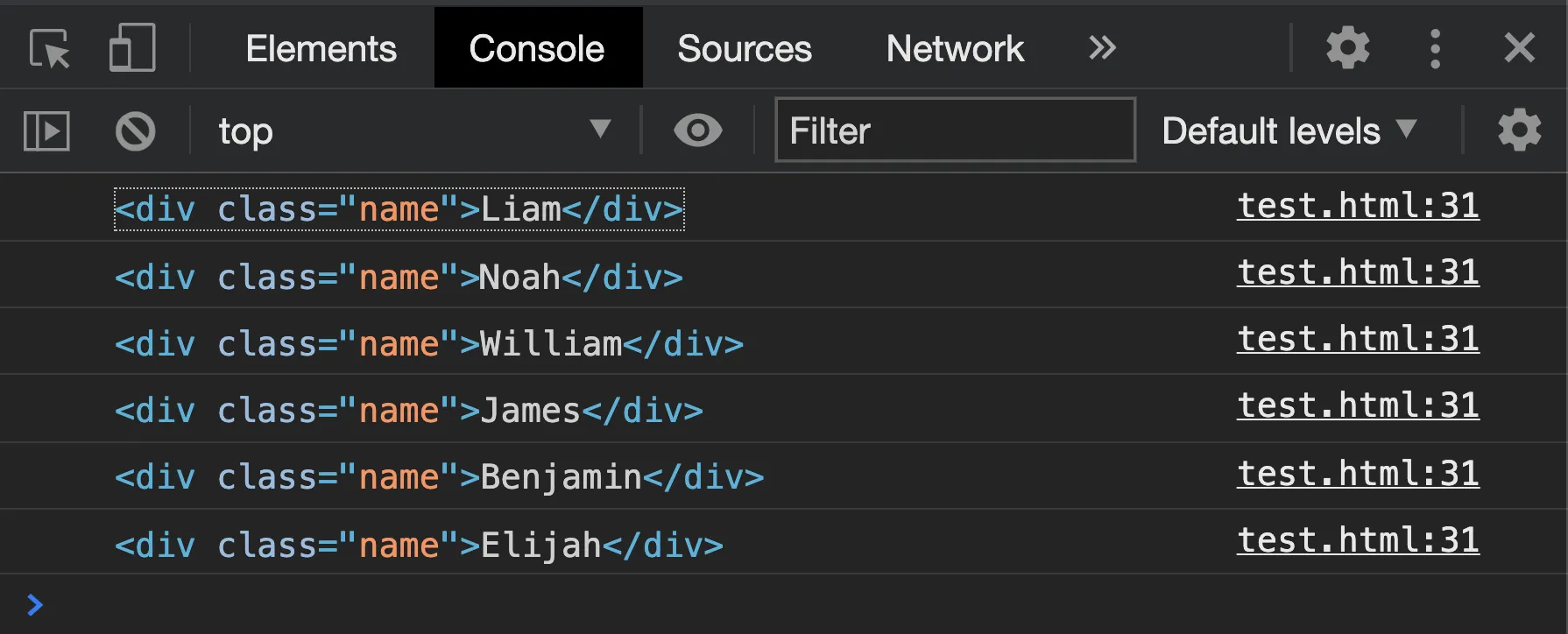
forEach Loop on NodeLists with querySelectorAll and Full Browser Support
The following code, unlike the code in the previous section, will work in all modern browsers:
let names = document.querySelectorAll('.name');
[].forEach.call(names, function(div) {
div.style.color = "blue";
});
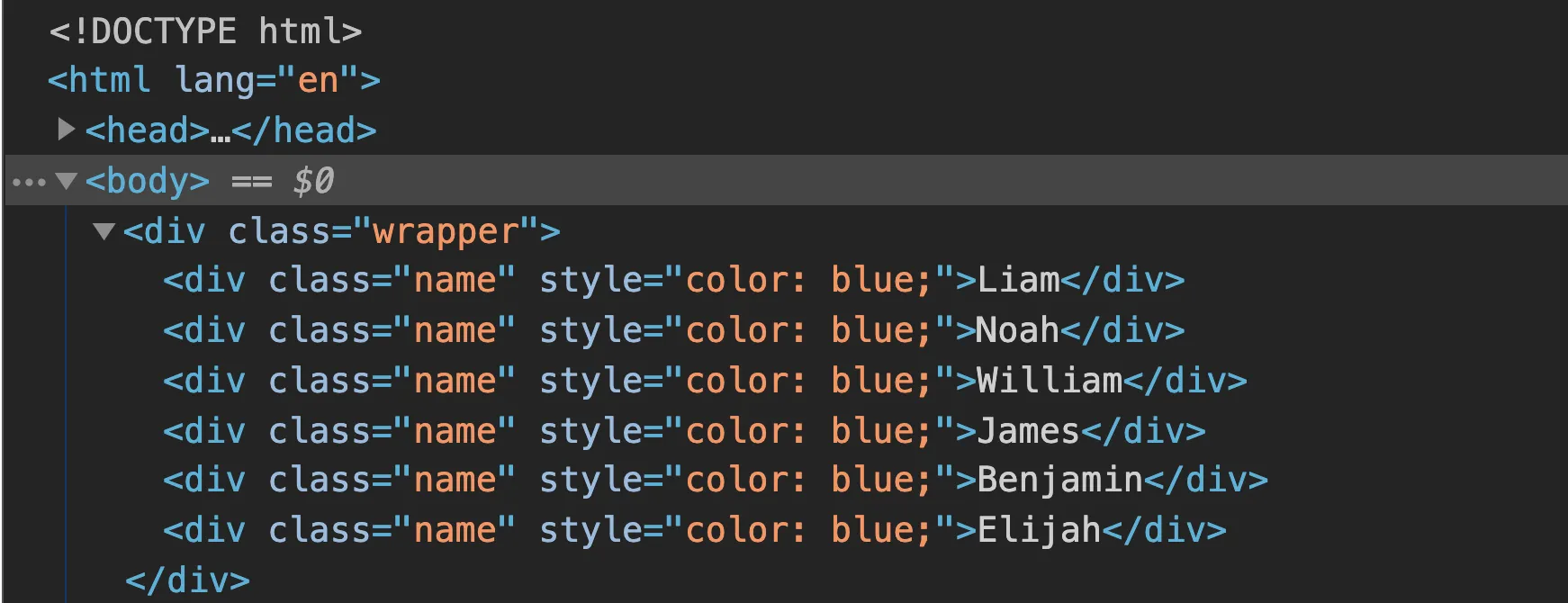
That’s it. Here are relevant posts on the topic: