The Document Object Model (DOM) is a key component in web development. It’s a programming interface for web documents which provides a structured representation of web pages as a tree.
The DOM represents the structure of a document as a tree of nodes, where each node corresponds to a part of the document (e.g., an element, attribute, or text). This model allows programming languages, like JavaScript, to manipulate the content, structure, and styling of web pages.
Further sections will cover the DOM’s structure, its integration with JavaScript, and essential methods for interactive web document manipulation.
The DOM was designed to be independent of any specific programming language, making it accessible through interfaces that languages like JavaScript can use.
What is DOM? Understanding the DOM Structure
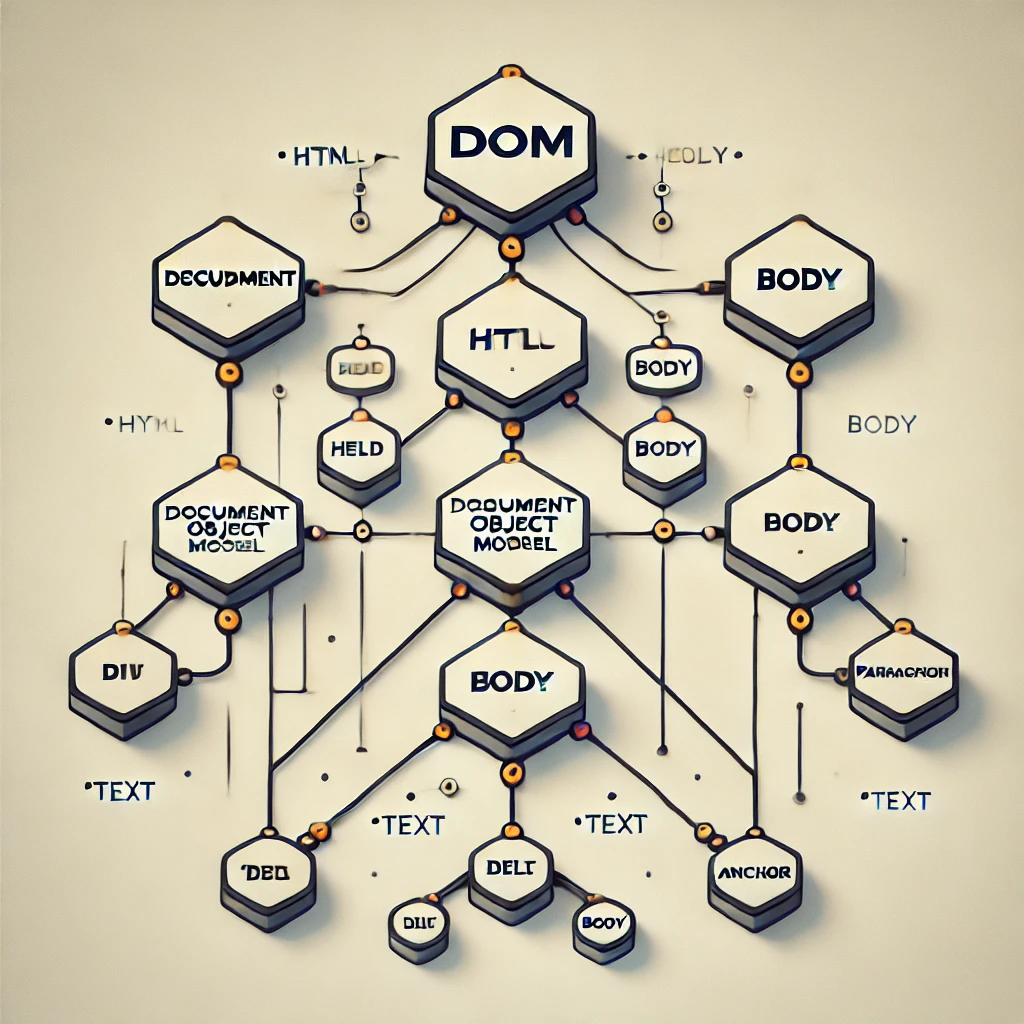
The Document Object Model (DOM) organizes a web document as a tree of nodes, making it easy to navigate and manipulate using programming languages like JavaScript.
Key Node Types
- Document Node: The root of the tree, representing the whole document.
- Element Nodes: Represent HTML or XML elements and can contain other element nodes, text nodes, and attributes.
- Text Nodes: Contain text content of elements and are always leaf nodes, meaning they cannot have child nodes.
- Attribute Nodes: Associated with element nodes but not considered children; they define characteristics such as id or class.
- Comment Nodes: Represent comments within the HTML or XML files, visible in the DOM tree but not rendered in the user interface.
Tree Structure
The DOM tree’s hierarchical structure includes parent, child, and sibling relationships:
- Parent Nodes: Nodes with child nodes under them in the tree.
- Child Nodes: Nodes directly nested within another node. For example, an
<li>
element inside an<ul>
. - Sibling Nodes: Nodes that share the same parent. For instance, two
<div>
elements within the same<body>
element are siblings.
This structured approach allows developers to efficiently target and manipulate elements, enabling dynamic updates and interactions on web pages.
Differences Between HTML DOM and DOM
Although “HTML DOM” and “DOM” are often used interchangeably, they serve distinct purposes in web development.
While both provide ways to interact with a document, the HTML DOM is specifically designed for HTML documents, offering specialized tools and methods, whereas the DOM provides a more generalized structure for interacting with any type of document, such as XML or HTML.
HTML DOM
The HTML DOM is a specialized version of the DOM tailored for HTML documents. It provides specific methods and properties like getElementById()
and innerHTML
for interacting with HTML elements and events.
DOM
The DOM (Document Object Model) is a broader interface applicable to various document types, including HTML and XML. It offers a generic way to access and manipulate document content but lacks HTML-specific features.
Key Differences
- The HTML DOM is a subset of the DOM, designed specifically for HTML documents.
- DOM is generic, while HTML DOM adds methods tailored to HTML manipulation.
Note: The HTML DOM extends the DOM, providing more tools for web development.
DOM and JavaScript
JavaScript interacts with the DOM to create dynamic web pages. By using JavaScript, developers can access and manipulate the DOM to update the content, style, and structure of a web page without requiring a full page reload. This interaction is what enables the creation of responsive and interactive web applications.
Accessing the DOM
Accessing the DOM is straightforward with JavaScript. You can use various methods provided by the DOM API to select and manipulate elements:
// Select an element by its ID
const element = document.getElementById('myElement');
// Select elements by their class name
const elements = document.getElementsByClassName('myClass');
// Select elements by their tag name
const tags = document.getElementsByTagName('div');
// Select elements using a CSS selector
const selectedElement = document.querySelector('.myClass');
// Select multiple elements using a CSS selector
const selectedElements = document.querySelectorAll('.myClass');
Fundamental Data Types
The DOM uses various data types to represent different parts of a document:
- Document: Represents the entire HTML or XML document.
- Element: Represents an element in the document (e.g.,
<div>
,<p>
). - Attribute: Represents an attribute of an element (e.g.,
class
,id
). - Text: Represents the text content of an element.
- Node: Represents any single node in the DOM tree (elements, attributes, text, etc.).
DOM Interfaces
The DOM API provides various interfaces to interact with and manipulate the DOM:
- Document: The entry point to the DOM, representing the entire HTML or XML document.
- Element: Represents an element in the DOM and provides methods to manipulate it (e.g.,
getElementById
,getElementsByClassName
). - Node: Represents a single node in the DOM tree and provides methods for interacting with nodes (e.g., appendChild,
removeChild
). - Event: Represents events that can occur in the DOM, such as clicks, key presses, and more.
Examples
Here are five practical examples of interacting with the DOM using JavaScript:
Example 1: Changing the Content of an Element
<div id="content">Original Content</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
const contentDiv = document.getElementById('content');
contentDiv.textContent = 'Updated Content';
}
</script>
This example shows how to change the content of an element. When the button is clicked, the content inside the <div>
is updated to “Updated Content”.
Example 2: Adding a New Element to the DOM
<div id="container">
<p>This is the container.</p>
</div>
<button onclick="addElement()">Add Element</button>
<script>
function addElement() {
const container = document.getElementById('container');
const newElement = document.createElement('p');
newElement.textContent = 'This is a new paragraph.';
container.appendChild(newElement);
}
</script>
In this example, we dynamically add a new <p>
element with the text “This is a new paragraph” into the container when the button is clicked.
Example 3: Removing an Element from the DOM
<div id="container">
<p id="paragraph">This paragraph will be removed.</p>
</div>
<button onclick="removeElement()">Remove Element</button>
<script>
function removeElement() {
const paragraph = document.getElementById('paragraph');
paragraph.remove();
}
</script>
This example removes an element from the DOM. Clicking the button removes the <p>
element with the id “paragraph”.
Example 4: Replacing an Element in the DOM
<div id="container">
<p id="oldParagraph">This paragraph will be replaced.</p>
</div>
<button onclick="replaceElement()">Replace Element</button>
<script>
function replaceElement() {
const container = document.getElementById('container');
const oldParagraph = document.getElementById('oldParagraph');
const newParagraph = document.createElement('p');
newParagraph.textContent = 'This is the new paragraph.';
container.replaceChild(newParagraph, oldParagraph);
}
</script>
In this example, we replace an existing element with a new one. Clicking the button replaces the <p>
with id “oldParagraph” with a new paragraph element.
Example 5: Cloning and Appending an Element
<div id="container">
<p id="paragraph">This paragraph will be cloned.</p>
</div>
<button onclick="cloneElement()">Clone Element</button>
<script>
function cloneElement() {
const container = document.getElementById('container');
const paragraph = document.getElementById('paragraph');
const clonedParagraph = paragraph.cloneNode(true);
container.appendChild(clonedParagraph);
}
</script>
This example demonstrates how to clone an element. Clicking the button creates a copy of the <p>
element and appends it to the same container.
Interacting with the DOM using Developer Tools
Modern web browsers come equipped with powerful developer tools that allow you to inspect, modify, and debug your HTML, CSS, and JavaScript in real-time. These tools provide a way to directly interact with the DOM, making it easier to understand how the DOM is structured and how it changes dynamically with JavaScript.
1. Inspecting the DOM
In most browsers, you can right-click on any part of the web page and select “Inspect” or press F12
to open the developer tools. The “Elements” tab in the developer tools displays the DOM tree for the current page, showing all HTML elements, attributes, and their nesting structure.
You can also hover over different elements in the “Elements” panel to see which part of the page they correspond to. This allows you to inspect the properties of any element, such as its attributes, styles, and event listeners.
2. Modifying the DOM
The developer tools allow you to directly edit the DOM without altering the actual source code. By selecting an element in the “Elements” tab, you can:
- Right-click and select “Edit as HTML” to change the element’s structure.
- Double-click on attributes or text content to edit them inline.
These changes are reflected immediately in the web page, but they only affect your local view and will be lost when the page is refreshed.
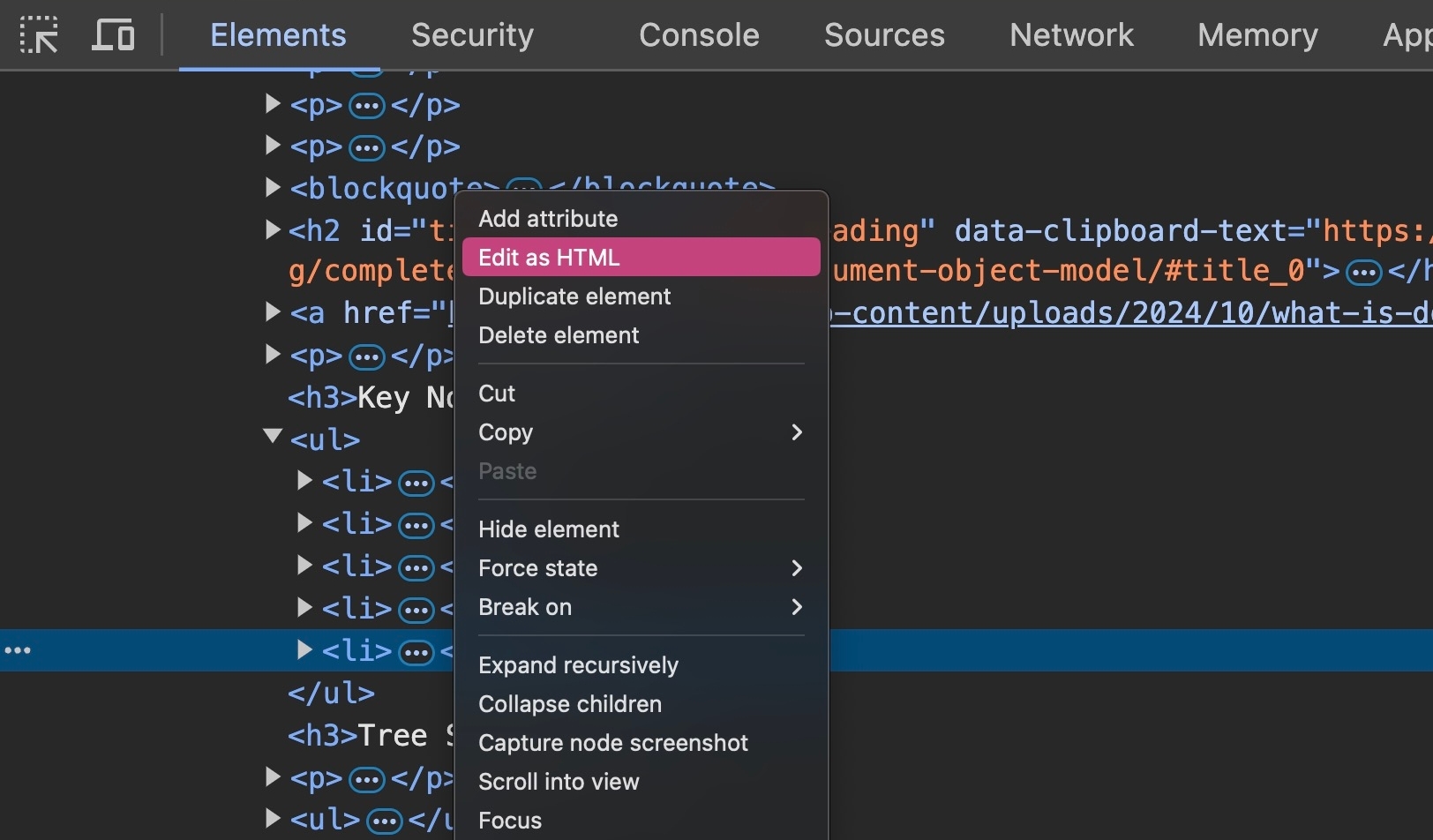
3. Viewing and Modifying CSS Styles
The developer tools also provide a “Styles” panel that shows the CSS rules applied to the selected DOM element. You can:
- Enable or disable individual CSS properties by toggling the checkboxes next to them.
- Add, edit, or remove CSS properties and see the changes in real-time.
This is useful for testing design adjustments without having to modify the actual CSS files.
4. Using the Console to Interact with the DOM
The “Console” tab allows you to run JavaScript commands to interact with the DOM directly. For example, you can retrieve elements, modify their content, or even add new elements.
Some common DOM interactions in the console include:
// Select an element by its ID
document.getElementById('myElement');
// Change the content of an element
document.getElementById('myElement').textContent = 'Updated content';
// Add a new class to an element
document.getElementById('myElement').classList.add('new-class');
// Remove an element from the DOM
document.getElementById('myElement').remove();
Using the console in developer tools is a quick and powerful way to test JavaScript code and immediately see the effect on the page.
5. Monitoring Events
Developer tools also allow you to monitor events happening in the DOM. You can:
- Right-click on an element in the “Elements” panel and select “Break on…” to pause JavaScript execution when certain changes occur, such as when an element is modified or removed.
- Use the “Event Listeners” panel to view all event listeners attached to an element, such as click or hover events.
This helps with debugging event-driven JavaScript applications.
Appendix:The DOM and PageSpeed Insights Warnings
PageSpeed Insights often flags large or inefficient DOM structures as potential performance issues. A large DOM can slow down rendering, increase memory usage, and cause sluggish interactions, especially on mobile devices.
This warning typically appears when the DOM has too many nodes or is deeply nested and would appear as Avoid Excessive DOM Size.
How to Address This Warning
- Minimize DOM Depth: Avoid deeply nested elements by using simpler HTML structures.
- Reduce Unnecessary Elements: Eliminate unnecessary
<div>
or<span>
tags that serve no functional or styling purpose. - Use Virtual DOM Techniques: In JavaScript frameworks, consider using a virtual DOM or batch DOM updates to improve performance.
Example: Simplifying a Deep DOM Structure
<!-- Inefficient DOM -->
<div class="outer">
<div class="inner">
<div class="content">
<p>Deeply nested structure</p>
</div>
</div>
</div>
<!-- Optimized DOM -->
<div class="content">
<p>Simplified structure</p>
</div>
By optimizing your DOM structure, you can reduce the likelihood of receiving a PageSpeed Insights warning related to DOM size and improve your site’s performance.
Here’s a comprehensive post on how to address Excessive DOM Size by Optimizing the DOM structure. It explains how to reduce the likelihood of receiving a warning from PageSpeed Insights related to DOM size and improve performance and page load time.
Conclusion
The Document Object Model (DOM) is an essential concept for web developers, enabling dynamic interaction with web documents. By understanding how to access and manipulate the DOM using JavaScript, you can create more interactive and responsive web applications.
The examples provided demonstrate basic operations such as changing content, adding elements, and removing elements, showcasing the power and flexibility of the DOM API.
Can I replace multiple DOM elements at once?
The DOM API allows you to replace one element at a time using the replaceChild() method. If you need to replace multiple elements at once, you can loop through the elements and replace them individually. Here’s an example:
Hope that helps…
Thanks for the quick reply! and if I want to replace multiple elements conditionally, like only replacing elements that contain specific text?
Pretty similar answer… you can loop through the elements and check their text content or any other condition before replacing them: