By default, WordPress does not show the featured image (thumbnail) in the list of posts in the admin panel. However, with a small code snippet, you can easily add a new column to display the post thumbnail next to the title, author, or date columns.
This tweak is especially helpful for editors and content managers who rely on visual cues to identify posts quickly.
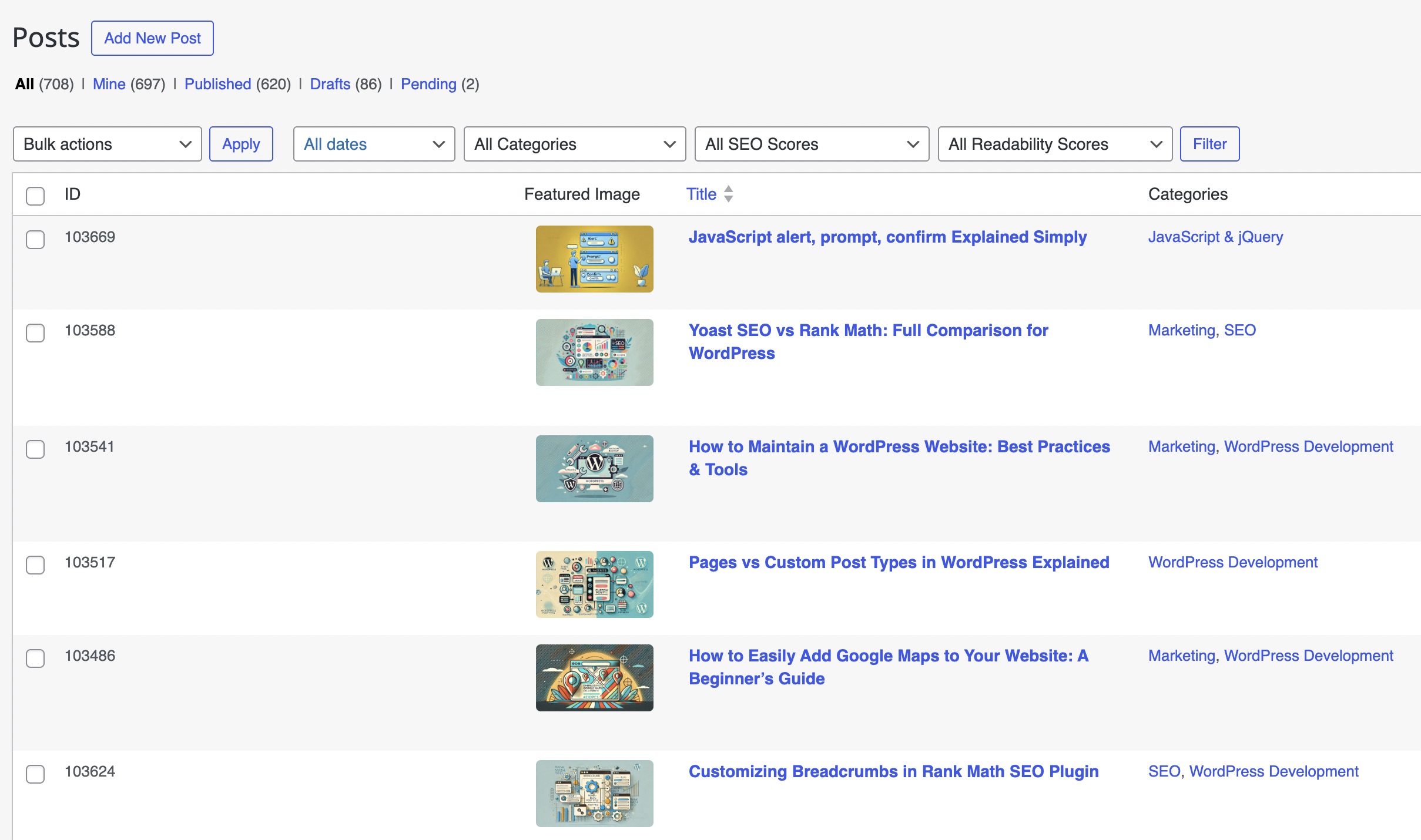
Add the Featured Image Column to the Admin Post List
To add the featured image column, you need to hook into two WordPress filters and one action: manage_posts_columns
, manage_posts_custom_column
, and admin_head
to style it.
// Add a new column to the posts list
add_filter('manage_posts_columns', 'add_featured_image_column');
function add_featured_image_column($columns) {
$new = [];
foreach ($columns as $key => $value) {
if ($key === 'title') {
$new['featured_image'] = __('Featured Image');
}
$new[$key] = $value;
}
return $new;
}
// Display the featured image in the custom column
add_action('manage_posts_custom_column', 'display_featured_image_column', 10, 2);
function display_featured_image_column($column, $post_id) {
if ($column === 'featured_image') {
$thumbnail = get_the_post_thumbnail($post_id, [60, 60]);
echo $thumbnail ?: '–';
}
}
// Optional: Style the column width
add_action('admin_head', 'featured_image_column_style');
function featured_image_column_style() {
echo '<style>
.column-featured_image { width: 80px; text-align: center; }
.column-featured_image img { border-radius: 4px; }
</style>';
}
Change the image size
You can control the size of the thumbnail displayed in the admin column by adjusting the parameters inside the get_the_post_thumbnail()
function.
The second argument accepts either a registered image size (like 'thumbnail'
, 'medium'
, 'custom-size'
) or an array of custom dimensions.
For example, to display a 100×100 image instead of 60×60, update this line:
$thumbnail = get_the_post_thumbnail($post_id, [100, 100]);
If you want to use a registered image size from your theme (like 'admin-thumb'
), you can also do:
$thumbnail = get_the_post_thumbnail($post_id, 'admin-thumb');
This gives you full flexibility over how large or small the image appears in the admin post list.
Where to Place the Code
You can place the above code in your theme’s functions.php
file or within a site-specific plugin. If you’re building a custom plugin for admin tweaks, this is a great candidate.
Avoid adding this to a parent theme if you’re not using a child theme, or you’ll lose it on the next update.
Make It Work for Custom Post Types
If you want to apply the same logic to a custom post type (e.g., portfolio
), replace the filters with their CPT versions:
// Replace 'portfolio' with your CPT slug
add_filter('manage_portfolio_posts_columns', 'add_featured_image_column');
add_action('manage_portfolio_posts_custom_column', 'display_featured_image_column', 10, 2);
Final Thoughts
This small enhancement can dramatically improve content management, especially for image-heavy sites or portfolios. By using native WordPress hooks, you ensure that your customization is fast, efficient, and doesn’t rely on extra plugins.
Want to display post IDs in the admin too? Check out our guide on showing post IDs in the WordPress dashboard without a plugin.