clip-path is a very interesting CSS property that allows you to “clip” a certain part of elements such as SVG, images, and HTML.
The property creates a shape that determines which part of the element will be visible. The part inside the shape will be visible, and the part outside of it will be hidden.
Before we explain more about the property, take a look at the following examples when you hover over them with the mouse. These can be done using clip-path
along with the anime.js library which I wrote a lot about:
In this post, we won’t explain how the above animations were done, you are welcome to take a look at Codrops’ website and get an explanation and examples of how it was done.
<span>Syntax</span>
Here are the existing options for using the clip-path property, we won’t explain all of them but will focus on the built-in shapes (lines 17-20), yet we will see a number of examples and I am sure you will understand through them how to use this cool CSS property:
/* Keyword values */
clip-path: none;
/* values */
clip-path: url(resources.svg#c1);
/* values */
clip-path: margin-box;
clip-path: border-box;
clip-path: padding-box;
clip-path: content-box;
clip-path: fill-box;
clip-path: stroke-box;
clip-path: view-box;
/* values */
clip-path: inset(100px 50px);
clip-path: circle(50px at 0 100px);
clip-path: polygon(50% 0%, 100% 50%, 50% 100%, 0% 50%);
clip-path: path('M0.5,1 C0.5,1,0,0.7,0,0.3 A0.25,0.25,1,1,1,0.5,0.3 A0.25,0.25,1,1,1,1,0.3 C1,0.7,0.5,1,0.5,1 Z');
/* Box and shape values combined */
clip-path: padding-box circle(50px at 0 100px);
/* Global values */
clip-path: inherit;
clip-path: initial;
clip-path: unset;
Setting Basic Shapes Using clip-path
The clip-path
feature allows a simple way to clip (or cut) basic shapes by using the keywords polygon, circle, ellipse, or inset, which are part of the CSS exclusion module.
Polygon
Polygon is the most flexible shape of the possible shapes because it allows you to determine an unlimited number of points similar to SVG Path. The points you provide will be pairs of X and Y coordinates that can be in pixels and percentages among others.
Because it is the most flexible shape, it is also the most “complicated” to create and you might want to use such a tool or another to determine the points.
Let’s give several examples. The first one (from right to left) is the original image, after it an image with clip-path
in the shape of a triangle, after it a slightly more complex shape and the last in the shape of a star:
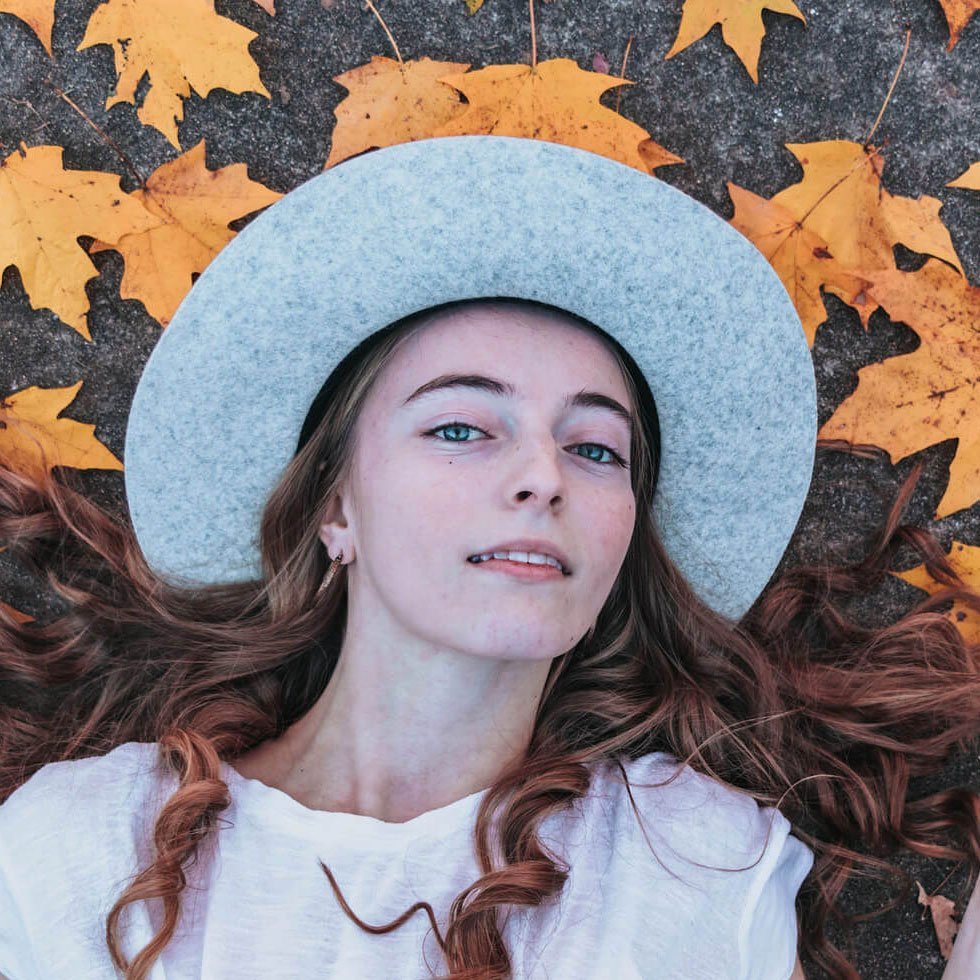
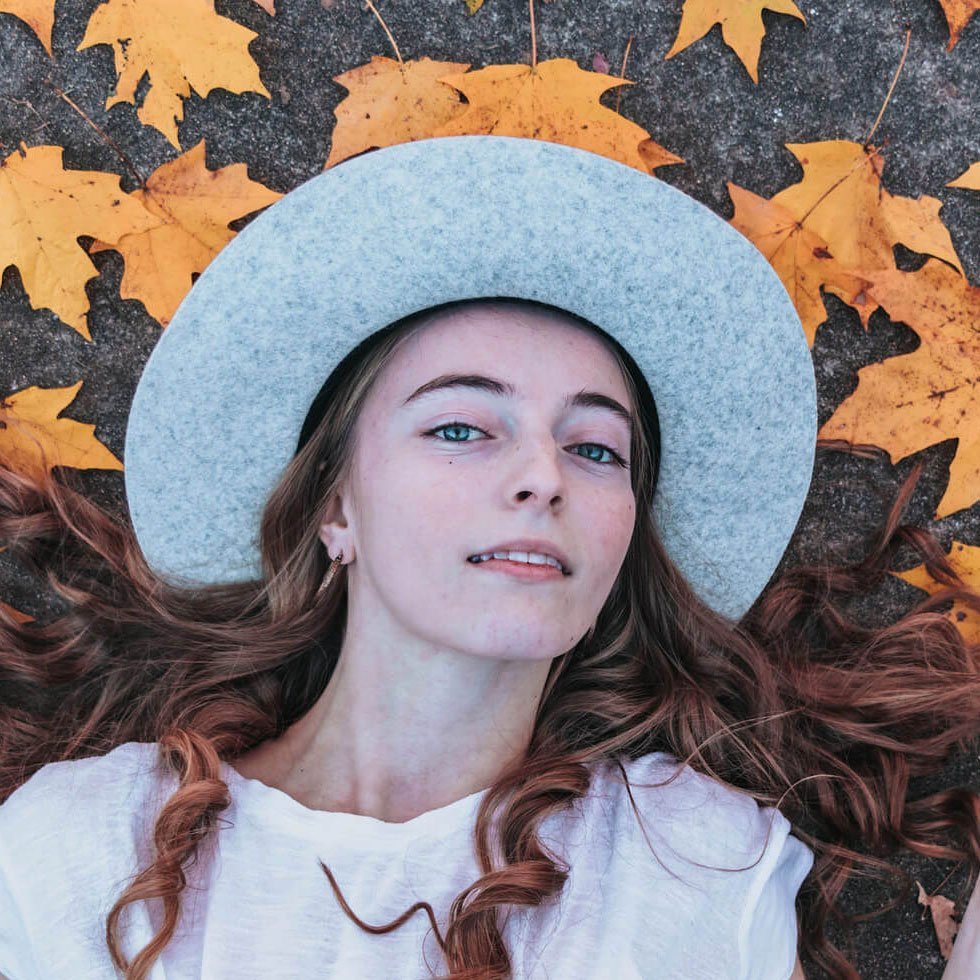
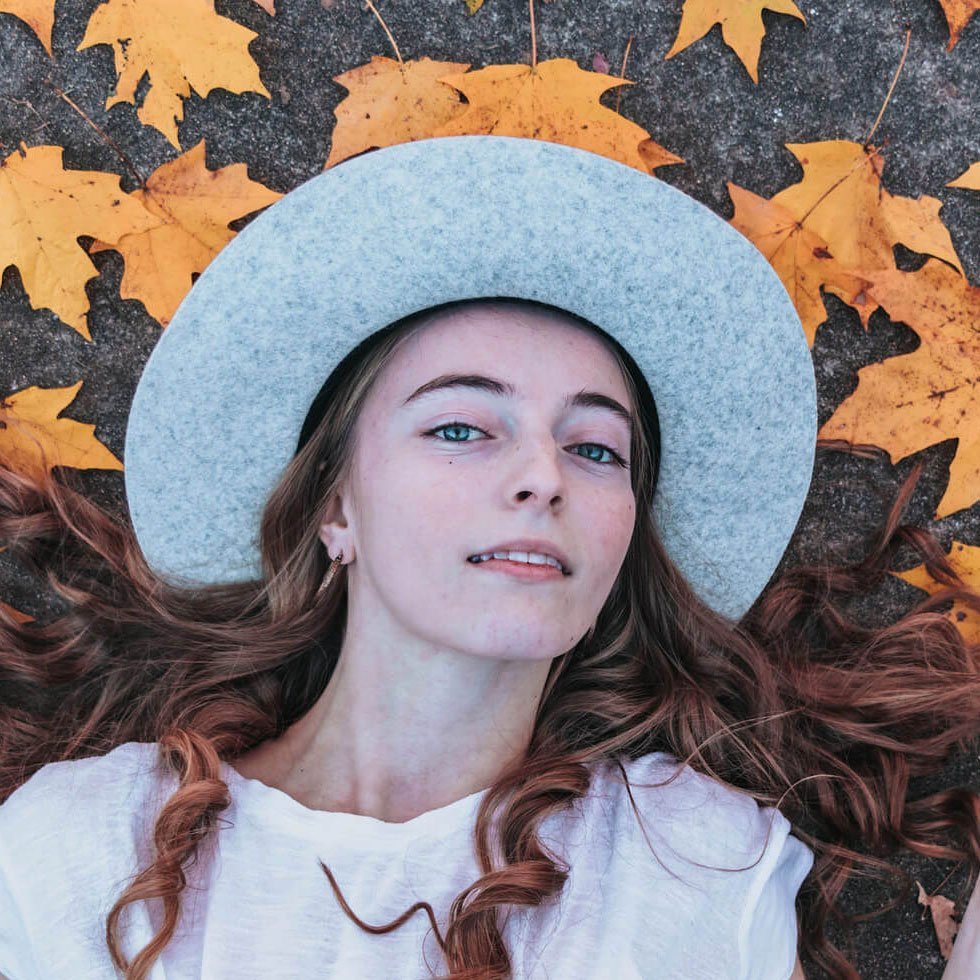
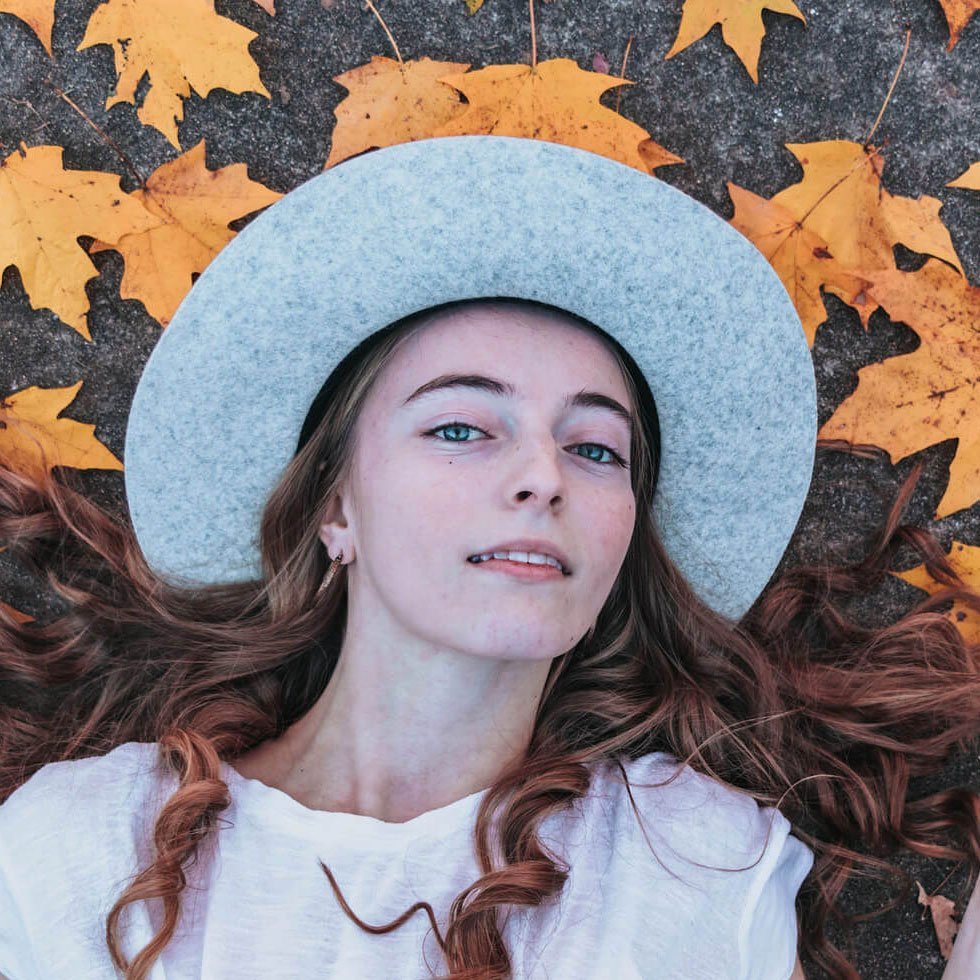
And this is the CSS we used:
.polygon1 {
-webkit-clip-path: polygon(50% 0%, 0% 100%, 100% 100%);
clip-path: polygon(50% 0%, 0% 100%, 100% 100%);
}
.polygon2 {
-webkit-clip-path: polygon(20% 0%, 0% 20%, 30% 50%, 0% 80%, 20% 100%, 50% 70%, 80% 100%, 100% 80%, 70% 50%, 100% 20%, 80% 0%, 50% 30%);
clip-path: polygon(20% 0%, 0% 20%, 30% 50%, 0% 80%, 20% 100%, 50% 70%, 80% 100%, 100% 80%, 70% 50%, 100% 20%, 80% 0%, 50% 30%);
}
.polygon3 {
-webkit-clip-path: polygon(50% 0%, 61% 35%, 98% 35%, 68% 57%, 79% 91%, 50% 70%, 21% 91%, 32% 57%, 2% 35%, 39% 35%);
clip-path: polygon(50% 0%, 61% 35%, 98% 35%, 68% 57%, 79% 91%, 50% 70%, 21% 91%, 32% 57%, 2% 35%, 39% 35%);
}
Circle
Circles in the clip-path
feature are defined using the following syntax:
circle(radius at posX posY)
Here are some examples:
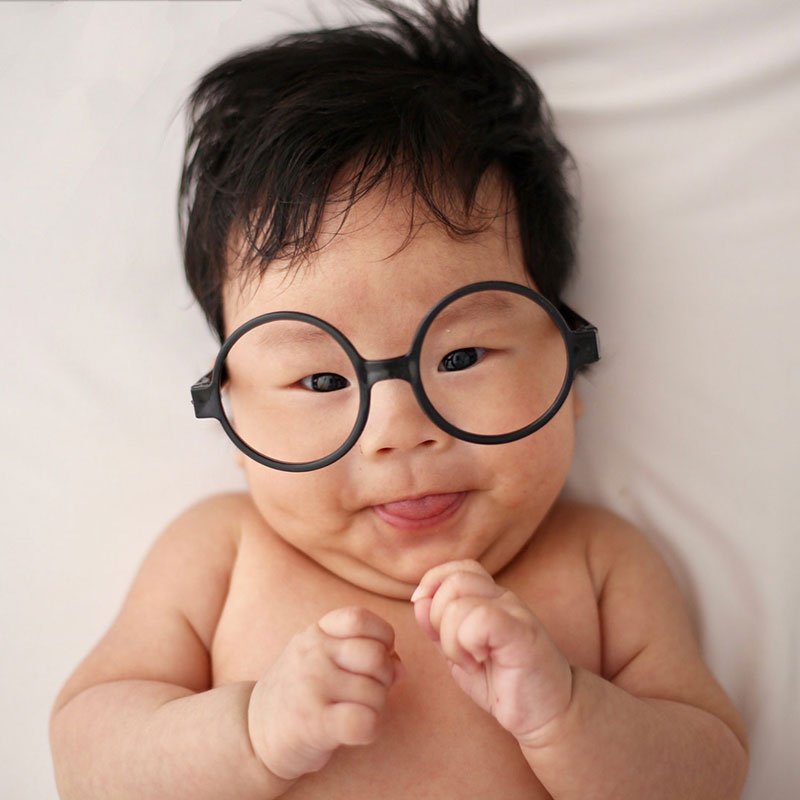
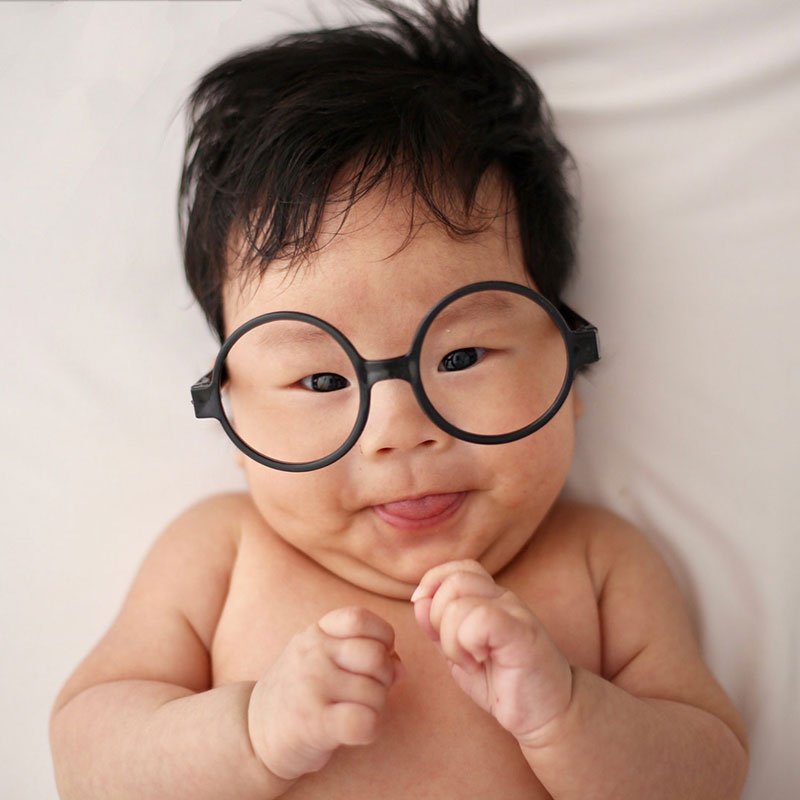
And this is the CSS we used:
.circle1 {
-webkit-clip-path: circle(50%);
clip-path: circle(50%);
}
.circle2 {
-webkit-clip-path: circle(70% at 70% 20%);
clip-path: circle(70% at 70% 20%);
}
Ellipse
An ellipse is defined using the following syntax:
ellipse(radiusX radiusY at posX posY)
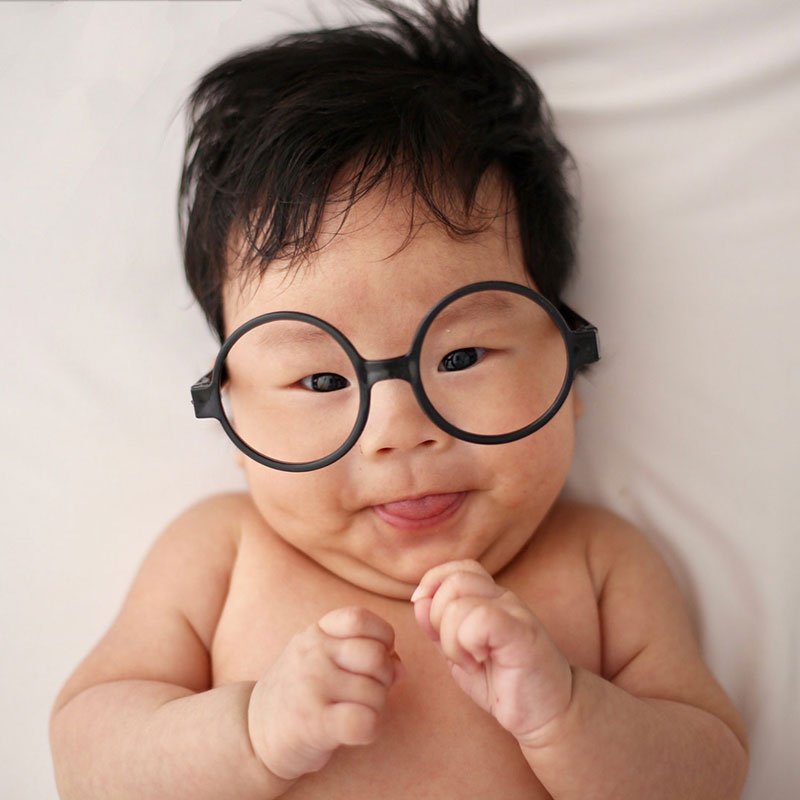
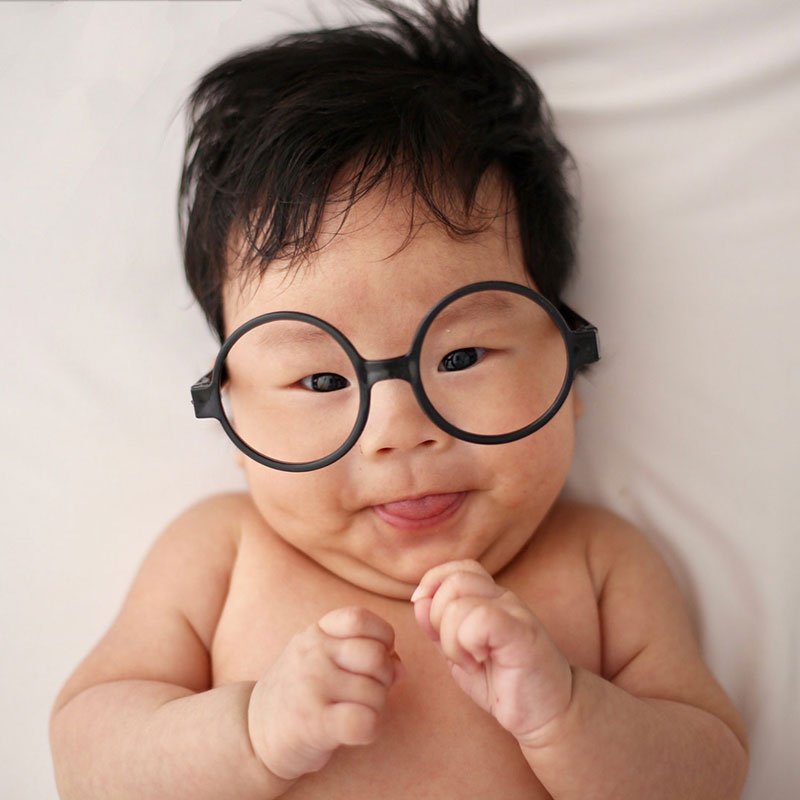
Here is the CSS we used:
.ellipse1 {
-webkit-clip-path: ellipse(50% 65% at 70% 50%);
clip-path: ellipse(50% 65% at 70% 50%);
}
.ellipse2 {
-webkit-clip-path: ellipse(50% 35%);
clip-path: ellipse(50% 35%);
}
Inset
Using inset, you can define an inner margin and everything outside this square will be clipped. You can also create a square with rounded edges using the keyword round and the radius value:
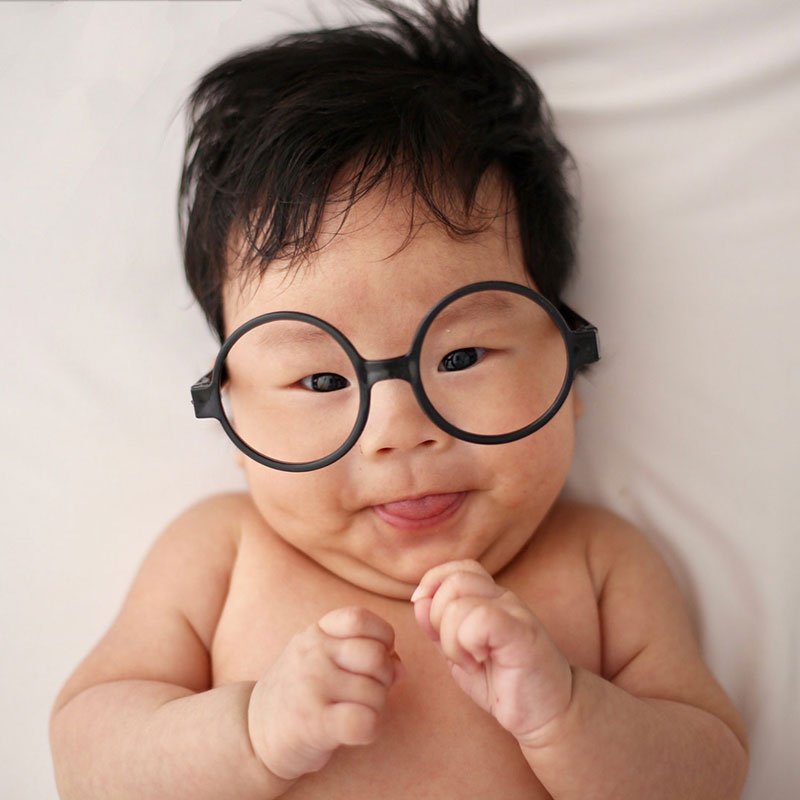
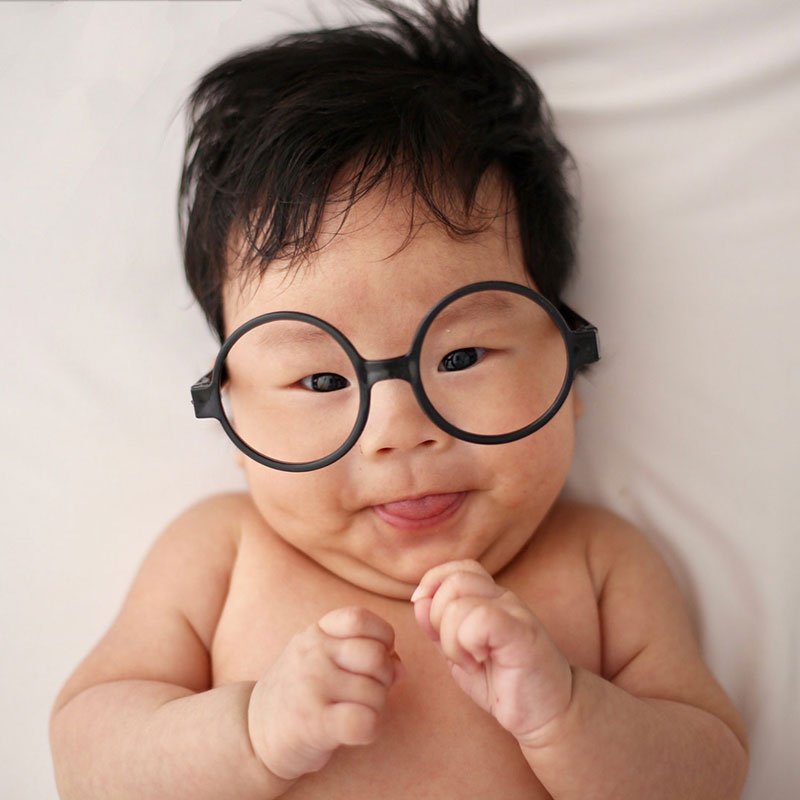
And this is the CSS we used:
.inset1 {
-webkit-clip-path: inset(20% 25% 20% 10%);
clip-path: inset(20% 25% 20% 10%);
}
.inset2 {
-webkit-clip-path: inset(35% 20% 41% 20% round 20px);
clip-path: inset(35% 20% 41% 20% round 20px);
}
Animations & Transitions
You can add animations to clip-path
to create cool and interesting effects. But you must ensure that every step of the animation contains the same number of points. Take a look:
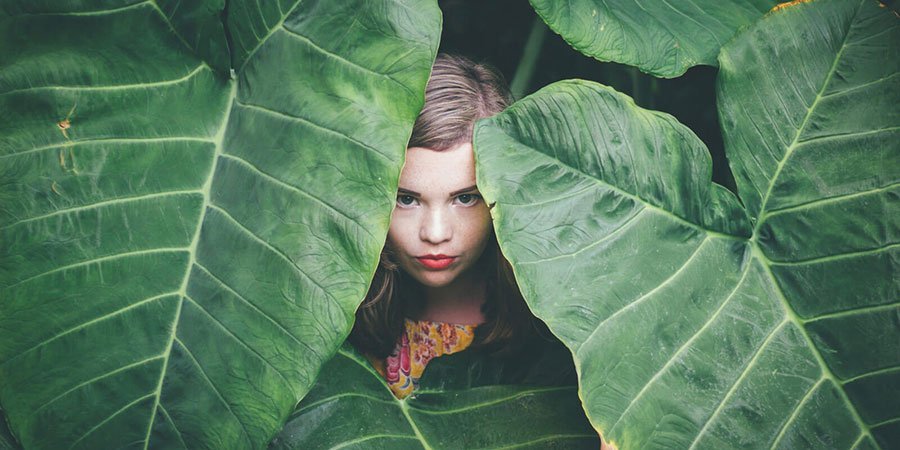
And here is the CSS we used to perform this animation:
.animate {
animation: shaping 4s infinite;
}
@keyframes shaping {
0% {
-webkit-clip-path: polygon(0 0, 100% 0, 100% 100%, 0% 100%);
clip-path: polygon(0 0, 100% 0, 100% 100%, 0% 100%);
}
20% {
-webkit-clip-path: polygon(28% 0, 73% 0, 100% 100%, 0% 100%);
clip-path: polygon(28% 0, 73% 0, 100% 100%, 0% 100%);
}
40% {
-webkit-clip-path: polygon(0 0, 100% 72%, 100% 100%, 0 35%);
clip-path: polygon(0 0, 100% 72%, 100% 100%, 0 35%);
}
60% {
-webkit-clip-path: polygon(50% 0, 50% 0, 100% 100%, 0% 100%);
clip-path: polygon(50% 0, 50% 0, 100% 100%, 0% 100%);
}
80% {
-webkit-clip-path: polygon(0 70%, 100% 0, 100% 32%, 0 100%);
clip-path: polygon(0 70%, 100% 0, 100% 32%, 0 100%);
}
100% {
-webkit-clip-path: polygon(0 0, 100% 0, 100% 100%, 0% 100%);
clip-path: polygon(0 0, 100% 0, 100% 100%, 0% 100%);
}
}
In Summary
Regarding browser support, it is recommended to use the prefix -webkit-
to cover the supporting browsers. Furthermore, it’s said that most browsers support most of the options of clip-pathֿ
, especially the basic shapes mentioned in this post.
I will soon write about Masking and SVG Masks, so stay tuned and subscribe to the mailing list! Comments and questions are welcome… 🙂