In JavaScript, objects are used to group related data and functions. These functions that belong to an object are known as methods. One important aspect of methods in JavaScript is the usage of the this
keyword, which often confuses beginners.
This guide will help you understand how methods work and how this
behaves in different contexts.
Object methods allow you to organize your code around real-world entities. The this keyword dynamically refers to the object that is calling the method.
1. Creating Object Methods
You can define a method inside an object by assigning a function to a property:
const user = {
name: "Alice",
greet: function() {
console.log("Hello, " + this.name + "!");
}
};
user.greet(); // Output: Hello, Alice!
In the example above, greet()
is a method of the user
object. It uses this.name
to access the name
property of the object.
2. Method Shorthand Syntax
JavaScript allows a shorter way to define methods inside objects:
const user = {
name: "Bob",
greet() {
console.log("Hello, " + this.name + "!");
}
};
user.greet(); // Output: Hello, Bob!
3. What is this?
The keyword this
refers to the object that is currently executing the function. When a method is called with dot notation, this
refers to the object before the dot.
const car = {
brand: "Toyota",
start() {
console.log(this.brand + " engine started.");
}
};
car.start(); // Output: Toyota engine started.
Remember: The value of
this
depends on how the function is called, not where it is defined.
4. Losing this Context
When a method is assigned to a variable or passed around, it can lose its original context.
const person = {
name: "Dana",
speak() {
console.log(this.name);
}
};
const sayName = person.speak;
sayName(); // Output: undefined (or error in strict mode)
To fix this, you can use bind()
to permanently bind this
to the original object:
const boundSpeak = person.speak.bind(person);
boundSpeak(); // Output: Dana
5. Arrow Functions and this
Arrow functions do not have their own this
; they inherit it from their surrounding scope. Be cautious when using them as methods.
const dog = {
name: "Rex",
bark: () => {
console.log(this.name); // 'this' does not refer to 'dog'
}
};
dog.bark(); // Output: undefined
Use regular functions for methods when you need to access this
correctly.
6. Visual Summary Table
Context | Value of this |
---|---|
Object method | The object |
Function (strict mode) | undefined |
Function (non-strict) | Global object (window) |
Arrow function | Lexical scope |
7. Common Pitfalls
- Using arrow functions for methods and expecting
this
to refer to the object. - Detaching methods from objects and losing their context.
- Assuming
this
always refers to the object where the function was defined.
8. Practical Use Case: To-Do Item
Here’s a more realistic example using a to-do item object:
const todo = {
title: "Learn JavaScript",
done: false,
markDone() {
this.done = true;
console.log(`"${this.title}" marked as done.`);
}
};
todo.markDone(); // Output: "Learn JavaScript" marked as done.
9. Try It in the Console
To better understand this
, open your browser’s developer tools (F12), go to the Console tab, and paste any of the code examples. You may notice that after executing a function like user.greet()
, the console outputs the expected message followed by undefined
.
This happens because the method does not explicitly return a value — the console displays the return value of the last executed expression, and in this case, it’s undefined
. This is normal and not an error.
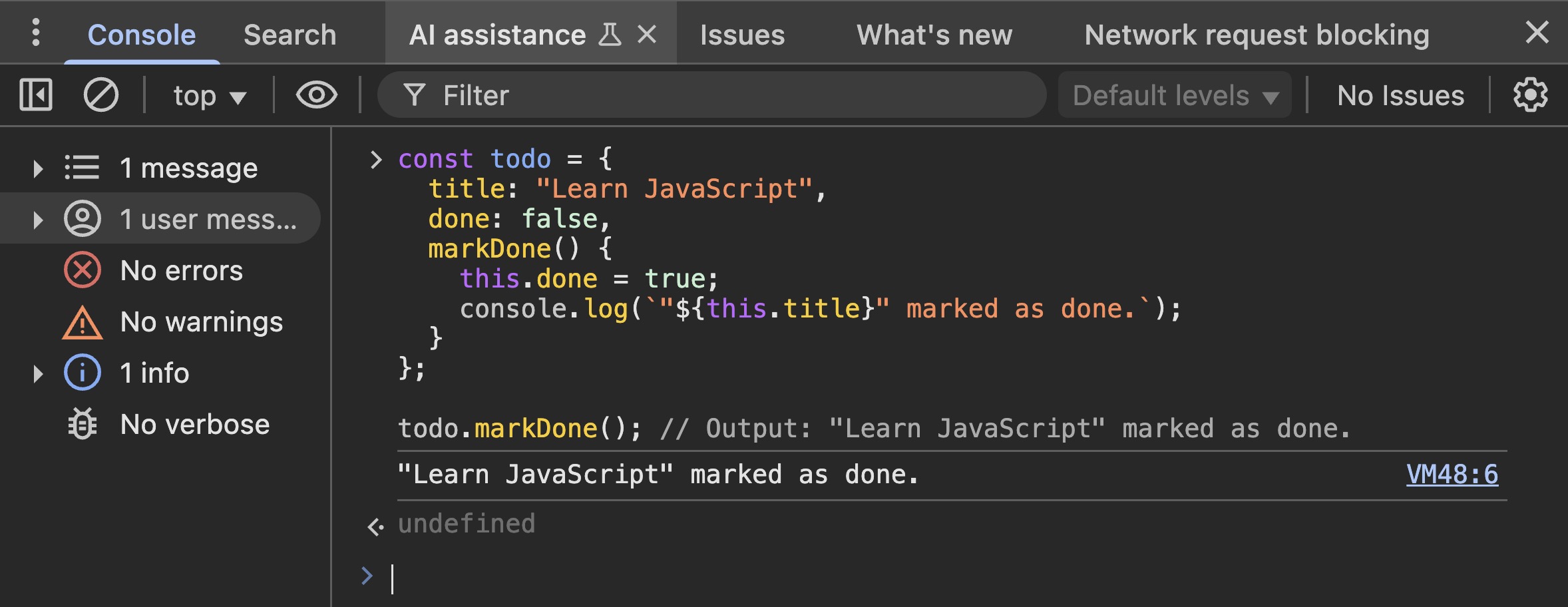
10. Using Strict Mode
Enabling strict mode changes the default behavior of this
in standalone functions. In strict mode, this
will be undefined
instead of referencing the global object.
"use strict";
function testThis() {
console.log(this); // undefined
}
testThis();
Real-World Example: User Management
Here’s how object methods and this
can be used in a simple user system:
const user = {
username: "admin",
login() {
console.log(this.username + " has logged in.");
},
logout() {
console.log(this.username + " has logged out.");
}
};
user.login(); // admin has logged in.
user.logout(); // admin has logged out.
This example shows how methods can represent actions and how
this
refers to the object that calls them.
Conclusion
Understanding object methods and the this
keyword is essential for mastering JavaScript. While it may be tricky at first, practice and experimentation will help solidify your understanding.
Keep experimenting with different object structures and calling contexts to see how this
behaves. As your skills grow, this concept will become second nature.