console.log()
is a fundamental function that plays a crucial role in debugging and monitoring code execution in JavaScript development. It allows developers to print messages, variable values, and other important information directly to the browser’s console.
This visibility into the code’s behavior helps in understanding issues more quickly and can significantly enhance your ability to diagnose and resolve problems in your code
In this guide i will cover various aspects of using console.log()
, including basic usage, advanced techniques, and best practices.
Basic Usage of console.log()
The most basic use of the console.log()
function is to print values to the console. This can include text, numbers, arrays, and objects:
console.log('Hello, World!');
console.log(42);
console.log([1, 2, 3]);
console.log({ name: 'Alice', age: 30 });
Here’s how it looks in Chrome developer console:
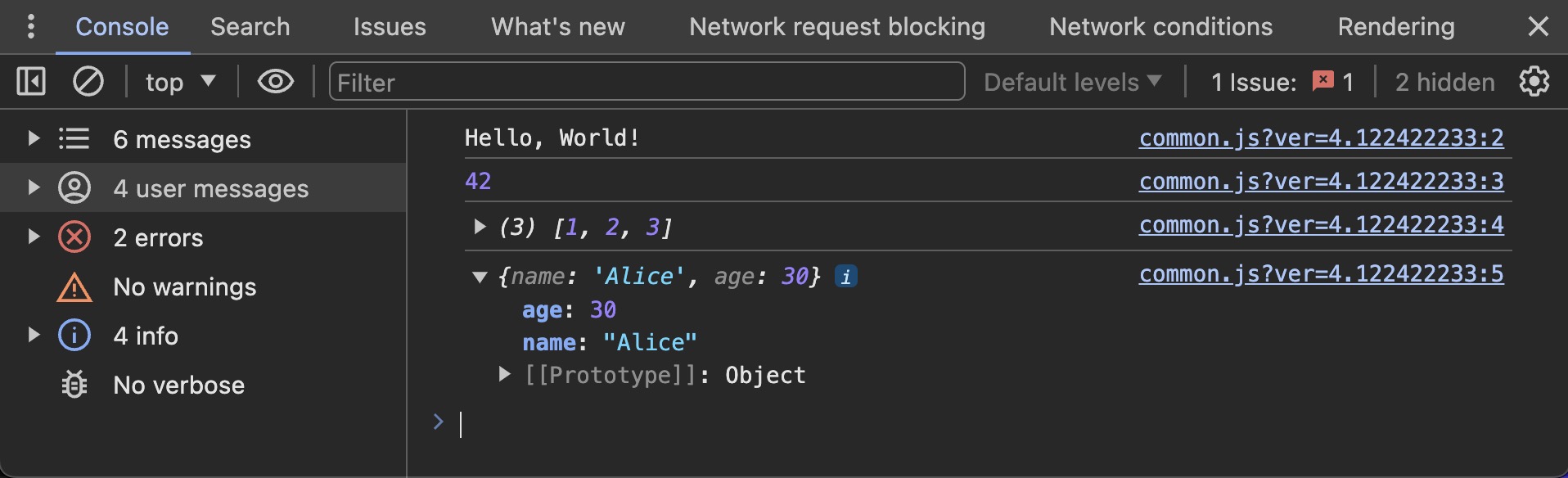
Using console.log() for Debugging
The console.log()
function is often used to debug JavaScript code. By printing variable values and expressions at different points in the code, you can trace the flow of execution and identify where things might be going wrong:
let x = 10;
let y = 20;
console.log('x:', x);
console.log('y:', y);
let z = x + y;
console.log('z:', z);
This helps in understanding the state of variables at different stages of the program.
Printing Multiple Values
You can print multiple values in a single console.log()
statement by separating them with commas:
let name = 'Bob';
let age = 25;
console.log('Name:', name, 'Age:', age);
Formatting Console Output
The console in many browsers supports string substitution, which allows for formatted output. Use %s
for strings, %d
for numbers, and %c
for CSS formatting:
console.log('Name: %s, Age: %d', 'Alice', 30);
console.log('%cThis is a styled message', 'color: blue; font-size: 20px;');
Formatted console output can make your debugging process more readable and organized.
Additional Example
Here is another example demonstrating the use of different placeholders and CSS formatting:
let user = 'John';
let score = 95;
console.log('User: %s, Score: %d', user, score);
console.log('%cWarning: High Score!', 'color: red; font-weight: bold;');
Viewing Objects and Arrays
When dealing with objects and arrays, console.log()
can be used to print and inspect their structure and content:
let person = { name: 'Charlie', age: 35 };
let numbers = [1, 2, 3, 4, 5];
console.log(person);
console.log(numbers);
Some browsers also support console.table()
for displaying arrays or objects as tables:
console.table(numbers);
console.table([person]);
Conditional Logging
You can use conditions to control logging, ensuring that certain messages are only logged when specific conditions are met:
let debug = true;
if (debug) {
console.log('Debug mode is on');
}
Conclusion
The console.log()
function is a versatile and powerful tool for JavaScript developers. It helps with debugging, tracking variable values, and understanding the flow of code execution. By mastering console.log()
, you can significantly improve your debugging and development workflow.